python如何实现word2vec
时间: 2023-10-02 20:05:35 浏览: 221
在Python中,可以使用gensim库来实现word2vec模型。下面是一个简单的示例代码:
首先,需要安装gensim库:
```
pip install gensim
```
然后,可以使用如下代码来训练word2vec模型:
```python
from gensim.models import Word2Vec
# 定义一个包含句子的列表,每个句子又是一个单词列表
sentences = [["this", "is", "a", "sentence"], ["another", "sentence"]]
# 训练word2vec模型
model = Word2Vec(sentences, size=100, window=5, min_count=1, workers=4)
# 查找与单词“sentence”最相似的10个单词
similar_words = model.wv.most_similar("sentence", topn=10)
print(similar_words)
```
上述代码中,`sentences`变量包含多个句子,每个句子又是一个单词列表。`Word2Vec`类用于训练word2vec模型,其中`size`参数指定了生成的词向量的维度,`window`参数指定了上下文窗口的大小,`min_count`参数指定了最小词频,`workers`参数指定了训练时使用的线程数。
在训练完成后,可以使用`most_similar`方法来查找与指定单词最相似的单词,其中`topn`参数指定了返回的最相似单词的数量。
相关问题
python实现word2vec
在Python中实现Word2Vec,可以使用gensim库。以下是一个简单示例:
```python
from gensim.models import Word2Vec
# 定义语料库
sentences = [['this', 'is', 'the', 'first', 'sentence', 'for', 'word2vec'],
['this', 'is', 'the', 'second', 'sentence'],
['yet', 'another', 'sentence'],
['one', 'more', 'sentence'],
['and', 'the', 'final', 'sentence']]
# 训练模型
model = Word2Vec(sentences, min_count=1)
# 打印和单词“sentence”最相似的单词
print(model.wv.most_similar('sentence'))
```
在这个示例中,我们定义了一个包含5个句子的语料库,并使用Word2Vec模型进行训练。然后,我们打印与单词“sentence”最相似的单词。
注意,我们使用了`min_count`参数,其默认值为5。这意味着只有在语料库中出现至少5次的单词才会被考虑。您可以根据您的需要调整此参数。
此外,Word2Vec模型还具有许多其他参数,例如`size`(单词向量的大小)和`window`(定义上下文窗口的大小)。您可以通过查看gensim文档来了解更多信息。
python实现word2vec词向量转化
要实现 word2vec 词向量转化,需要使用 Python 中的 gensim 库。下面是一些示例代码:
1. 导入 gensim 库
```python
import gensim
```
2. 加载语料库
```python
sentences = [["this", "is", "a", "sentence"], ["this", "is", "another", "sentence"]]
model = gensim.models.Word2Vec(sentences, min_count=1)
```
3. 查看词向量
```python
vector = model.wv['sentence']
print(vector)
```
这将输出一个 100 维的词向量数组。
4. 查找相似词
```python
similar_words = model.wv.most_similar('sentence')
print(similar_words)
```
这将输出与 "sentence" 最相似的 10 个单词及其相似度分数。
以上是一个基本的 word2vec 词向量转化的实现,可以根据需要进行适当的修改和扩展。
阅读全文
相关推荐
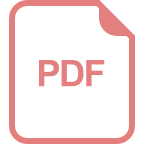
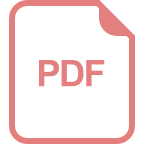
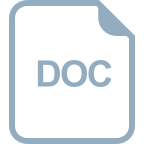
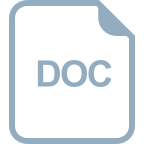
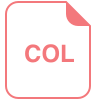






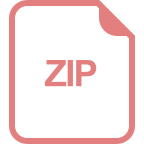
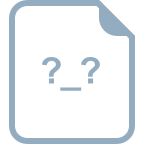
