opencv通过傅里叶变换去除图像规律噪声的c++代码
时间: 2023-10-09 18:08:24 浏览: 85
以下是使用OpenCV的傅里叶变换去除图像规律噪声的C ++代码示例:
```
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat image = imread("input.jpg", IMREAD_GRAYSCALE);
if (image.empty())
{
std::cout << "Could not open or find the image" << std::endl;
return -1;
}
// 将图像扩展到最佳的大小
Mat padded;
int m = getOptimalDFTSize(image.rows);
int n = getOptimalDFTSize(image.cols);
copyMakeBorder(image, padded, 0, m - image.rows, 0, n - image.cols, BORDER_CONSTANT, Scalar::all(0));
// 将图像转换为复数形式
Mat planes[] = { Mat_<float>(padded), Mat::zeros(padded.size(), CV_32F) };
Mat complexImage;
merge(planes, 2, complexImage);
// 执行傅里叶变换
dft(complexImage, complexImage);
// 创建高斯滤波器
Mat filter = Mat(padded.size(), CV_32F);
float sigma = 10;
float centerx = filter.cols / 2;
float centery = filter.rows / 2;
for (int i = 0; i < filter.rows; i++)
{
for (int j = 0; j < filter.cols; j++)
{
float distance = (j - centerx)*(j - centerx) + (i - centery)*(i - centery);
filter.at<float>(i, j) = exp(-distance / (2 * sigma*sigma));
}
}
// 将滤波器应用于频域图像
Mat planes2[] = { Mat::zeros(complexImage.size(), CV_32F), Mat::zeros(complexImage.size(), CV_32F) };
split(complexImage, planes2);
planes2[0] = planes2[0].mul(filter);
planes2[1] = planes2[1].mul(filter);
merge(planes2, 2, complexImage);
// 执行傅里叶反变换
idft(complexImage, complexImage);
// 从复数形式转换为幅度图像
Mat restoredImage;
split(complexImage, planes);
magnitude(planes[0], planes[1], restoredImage);
// 将图像裁剪到原始大小
restoredImage = restoredImage(Rect(0, 0, image.cols, image.rows));
// 显示结果
imshow("原始图像", image);
imshow("去除规律噪声后的图像", restoredImage);
waitKey();
return 0;
}
```
在此示例中,我们首先读取输入灰度图像。然后,我们将图像扩展到最佳大小,这是执行傅里叶变换的要求。接下来,我们将图像转换为复数形式,并执行傅里叶变换。然后,我们创建高斯滤波器,并将其应用于频域图像。最后,我们执行傅里叶反变换,并将结果转换为幅度图像。最后,我们将图像裁剪回原始大小,并显示结果。
阅读全文
相关推荐







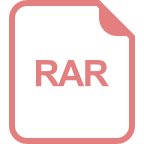
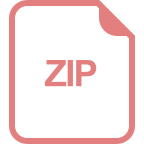
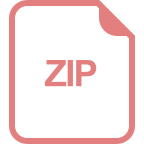
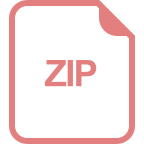
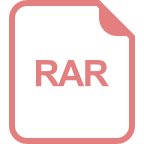