js开发opcua客户端代码
时间: 2023-07-24 21:33:22 浏览: 43
下面是一个使用opcua模块创建OPC UA客户端的示例代码:
```javascript
const opcua = require("node-opcua");
const endpointUrl = "opc.tcp://localhost:4840"; // OPC UA服务器的地址和端口号
// 创建一个OPC UA客户端对象
const client = new opcua.OPCUAClient();
(async () => {
try {
// 连接到OPC UA服务器
await client.connect(endpointUrl);
// 创建一个OPC UA会话对象
const session = await client.createSession();
// 读取一个变量节点的值
const nodeId = "ns=1;s=Temperature"; // 变量节点的ID
const dataValue = await session.read({ nodeId });
console.log("Temperature:", dataValue.value.value);
// 写入一个变量节点的值
const newValue = { dataType: opcua.DataType.Double, value: 25.0 }; // 新的值
await session.writeSingleNode(nodeId, newValue);
console.log("Temperature has been set to", newValue.value);
// 监控一个变量节点的值变化
const subscription = opcua.ClientSubscription.create(session, {
requestedPublishingInterval: 1000, // 发布间隔为1秒
requestedLifetimeCount: 1000,
requestedMaxKeepAliveCount: 10,
maxNotificationsPerPublish: 100,
publishingEnabled: true,
priority: 10,
});
subscription.on("started", () => {
console.log("Subscription started - monitoring Temperature");
});
const monitoredItem = await subscription.monitor({
nodeId,
attributeId: opcua.AttributeIds.Value,
dataEncoding: { namespaceIndex: 0, name: null },
});
monitoredItem.on("changed", (dataValue) => {
console.log("Temperature has changed:", dataValue.value.value);
});
// 等待一段时间后关闭会话和客户端
await new Promise((resolve) => setTimeout(resolve, 5000));
await subscription.terminate();
await session.close();
await client.disconnect();
} catch (err) {
console.log("Error:", err.message);
}
})();
```
这段代码使用了async/await语法,它通过Node.js的OPC UA客户端连接到了OPC UA服务器,并读取、写入和监控了一个变量节点的值。您可以根据自己的需要修改代码中的节点ID和值,以及订阅的间隔时间等参数。
相关推荐
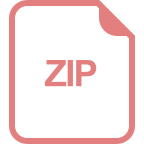
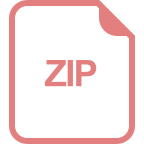
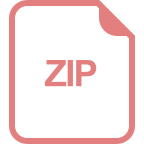
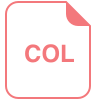
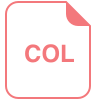
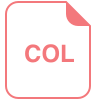
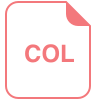
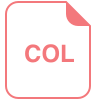









