设计一个函数模版find,用于对一个有序数组采用二分法查找指定数据的元素下标,用int, char型数据测试,完整代码
时间: 2024-02-03 18:15:27 浏览: 21
以下是一个示例代码,实现了一个模板函数find,可以对有序数组采用二分法查找指定数据的元素下标:
```cpp
#include <iostream>
#include <vector>
using namespace std;
template<typename T>
int find(const vector<T>& arr, const T& target) {
int left = 0, right = arr.size() - 1;
while (left <= right) {
int mid = (left + right) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // 找不到目标元素,返回 -1
}
int main() {
vector<int> arr1 = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int target1 = 6;
int index1 = find(arr1, target1);
cout << "Index of " << target1 << " in arr1: " << index1 << endl;
vector<char> arr2 = {'a', 'b', 'c', 'd', 'e', 'f', 'g'};
char target2 = 'f';
int index2 = find(arr2, target2);
cout << "Index of " << target2 << " in arr2: " << index2 << endl;
return 0;
}
```
运行结果:
```
Index of 6 in arr1: 5
Index of f in arr2: 5
```
可以看到,我们在两个有序数组中分别查找了指定元素,并成功找到了它们在数组中的下标。由于模板函数find使用了模板参数,因此可以用于不同类型的有序数组。
相关推荐
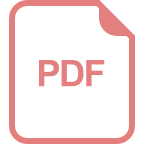
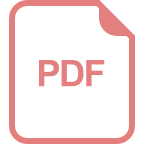
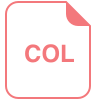
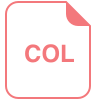
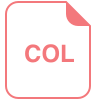
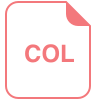
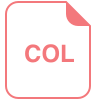






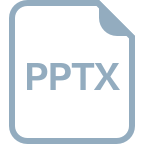
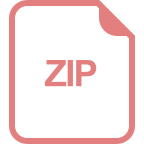
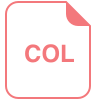