java byte 转GB工具类
时间: 2023-11-20 22:05:32 浏览: 148
以下是将byte转换为GB的Java工具类示例:
```java
public class ByteToGBUtil {
public static double convertToGB(long bytes) {
double gb = bytes / (1024.0 * 1024.0 * 1024.0);
return gb;
}
}
```
使用示例:
```java
long fileSize = 1234567890; //文件大小为1234567890字节
double gbSize = ByteToGBUtil.convertToGB(fileSize); //转换为GB
System.out.println(gbSize); //输出结果为1.1497805150146484
```
注意,由于计算机内存存储单位是2的幂次方,而不是10的幂次方,因此在将byte转换为GB时需要使用1024而不是1000作为进制。
相关问题
Java的Byte转MB工具类
你可以使用以下代码实现Byte转MB的工具类:
```java
public class ByteToMBConverter {
public static double convertBytesToMegabytes(long bytes) {
return (bytes / 1024d) / 1024d;
}
}
```
使用示例:
```java
long bytes = 2147483648L; // 2 GB
double megabytes = ByteToMBConverter.convertBytesToMegabytes(bytes);
System.out.println("2 GB in MB: " + megabytes);
```
输出结果为:2 GB in MB: 2048.0
java kb mb Gb转换工具类
好的,以下是Java中用于KB/MB/GB转换的工具类代码示例:
```
public class ConvertUtils {
private static final long KB = 1024;
private static final long MB = KB * 1024;
private static final long GB = MB * 1024;
public static String byteToReadable(long bytes) {
if (bytes >= GB) {
return String.format("%.2f GB", (float) bytes / GB);
} else if (bytes >= MB) {
return String.format("%.2f MB", (float) bytes / MB);
} else if (bytes >= KB) {
return String.format("%.2f KB", (float) bytes / KB);
} else {
return String.format("%d Bytes", bytes);
}
}
public static long toByte(String size) {
if (size == null || "".equals(size.trim())) {
return 0;
}
size = size.toUpperCase(Locale.ENGLISH);
String unit = "B";
if (size.endsWith("KB")) {
unit = "KB";
size = size.substring(0, size.length() - 2).trim();
} else if (size.endsWith("MB")) {
unit = "MB";
size = size.substring(0, size.length() - 2).trim();
} else if (size.endsWith("GB")) {
unit = "GB";
size = size.substring(0, size.length() - 2).trim();
} else if (size.endsWith("B")) {
size = size.substring(0, size.length() - 1).trim();
}
double value = Double.parseDouble(size);
if ("KB".equals(unit)) {
value *= KB;
} else if ("MB".equals(unit)) {
value *= MB;
} else if ("GB".equals(unit)) {
value *= GB;
}
return (long) value;
}
}
```
使用方法:
```java
long sizeInBytes = ConvertUtils.toByte("1GB");
String readableSize = ConvertUtils.byteToReadable(sizeInBytes);
System.out.println(readableSize); //expected output: 1.00 GB
```
希望这个工具类能够帮到您。
阅读全文
相关推荐
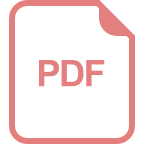
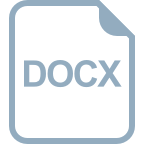
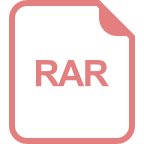
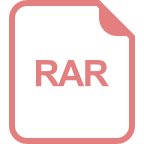
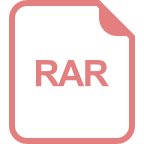
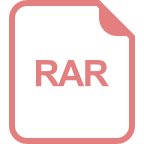
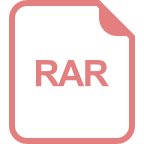
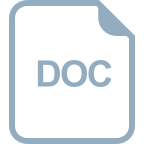
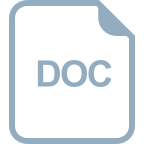
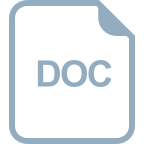
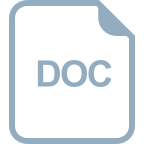
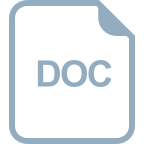
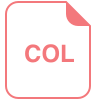
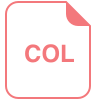