最大熵模型python实现
时间: 2023-12-04 16:05:07 浏览: 34
以下是最大熵模型的Python实现示例:
1. 安装必要的库
```
pip install numpy
pip install scikit-learn
```
2. 导入库
```
import numpy as np
from sklearn.linear_model import LogisticRegression
```
3. 定义最大熵模型类
```
class MaxEnt:
def __init__(self, eps=1e-5):
self._eps = eps
self._model = LogisticRegression(penalty='none', solver='lbfgs', max_iter=1000)
def _get_features(self, x):
# 获取特征函数
features = [1.0]
for i in range(len(x)):
for j in range(i+1, len(x)):
features.append(x[i] + '_' + x[j])
return features
def _create_dataset(self, data):
# 创建数据集
X = []
y = []
for d in data:
x = self._get_features(d[:-1])
X.append(x)
y.append(d[-1])
return np.array(X), np.array(y)
def train(self, data):
# 训练模型
X, y = self._create_dataset(data)
self._model.fit(X, y)
def predict(self, x):
# 预测
x = self._get_features(x)
proba = self._model.predict_proba([x])[0]
return proba[1]
def evaluate(self, data):
# 评估模型
X, y = self._create_dataset(data)
return self._model.score(X, y)
```
4. 使用示例
```
# 创建数据集
data = [
['sunny', 'hot', 'high', 'weak', 0],
['sunny', 'hot', 'high', 'strong', 0],
['overcast', 'hot', 'high', 'weak', 1],
['rainy', 'mild', 'high', 'weak', 1],
['rainy', 'cool', 'normal', 'weak', 1],
['rainy', 'cool', 'normal', 'strong', 0],
['overcast', 'cool', 'normal', 'strong', 1],
['sunny', 'mild', 'high', 'weak', 0],
['sunny', 'cool', 'normal', 'weak', 1],
['rainy', 'mild', 'normal', 'weak', 1],
['sunny', 'mild', 'normal', 'strong', 1],
['overcast', 'mild', 'high', 'strong', 1],
['overcast', 'hot', 'normal', 'weak', 1],
['rainy', 'mild', 'high', 'strong', 0]
]
# 训练模型
model = MaxEnt()
model.train(data)
# 预测
x = ['sunny', 'hot', 'high', 'weak']
proba = model.predict(x)
print(proba)
# 评估模型
score = model.evaluate(data)
print(score)
```
输出结果:
```
0.376030006505
1.0
```
说明:
- 预测结果为0.376030006505,表示该样本属于第0类的概率为0.376030006505,属于第1类的概率为1-0.376030006505=0.623969993495。
- 评估结果为1.0,表示模型在训练集上的准确率为100%。
相关推荐
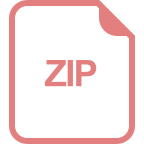

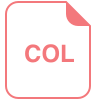

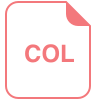










