基于最大熵原理的贝叶斯评定python 代码
时间: 2023-11-09 20:05:51 浏览: 39
下面是一个基于最大熵原理的贝叶斯分类器的 Python 代码,用于评估文本数据:
```python
import math
class MaxEnt:
def __init__(self):
self.feats = [] # 存放特征的列表
self.trainset = [] # 存放训练集的列表
self.labels = set() # 存放标签的集合
self.weights = [] # 存放权重的列表
def load_data(self, filename):
'''
加载数据集
'''
with open(filename, 'r') as f:
for line in f:
fields = line.strip().split()
# 第一个字段是标签,后面是特征
label = fields[0]
self.labels.add(label)
feats = {}
for field in fields[1:]:
feat, value = field.split(':')
feats[feat] = int(value)
self.trainset.append((feats, label))
def calc_empirical_expectation(self, feat):
'''
计算经验分布下的特征函数的期望
'''
count = 0
for feats, label in self.trainset:
if feat in feats:
count += 1
return float(count) / float(len(self.trainset))
def calc_model_expectation(self, feat):
'''
计算模型分布下的特征函数的期望
'''
count = 0.0
for label in self.labels:
weight = self.weights[label]
for feats, l in self.trainset:
if l != label:
continue
if feat in feats:
count += weight * feats[feat] / float(len(self.trainset))
return count
def train(self, max_iter=1000):
'''
训练模型
'''
self.feats = set()
for feats, label in self.trainset:
for feat in feats:
self.feats.add(feat)
for label in self.labels:
self.weights.append(0.0)
for i in range(max_iter):
print('Iter:%d' % i)
empirical_feats_count = [0.0] * len(self.feats)
model_feats_count = [0.0] * len(self.feats)
for feats, label in self.trainset:
for j, feat in enumerate(self.feats):
if feat in feats:
empirical_feats_count[j] += 1.0
# 计算 p(y|x)
prob = self.calc_prob(feats)
for j, feat in enumerate(self.feats):
if feat in feats:
model_feats_count[j] += prob[label] * feats[feat]
# 更新权重
for j, w in enumerate(self.weights):
delta = 1.0 / len(self.trainset) * math.log(self.calc_empirical_expectation(j) / self.calc_model_expectation(j))
self.weights[j] += delta
print(self.weights)
def calc_prob(self, feats):
'''
计算 p(y|x)
'''
features = {}
for feat in feats:
if feat not in self.feats:
continue
features[feat] = feats[feat]
prob = {}
Z = 0.0
for label in self.labels:
weight = self.weights[label]
feat_weight = 0.0
for feat in features:
feat_weight += features[feat] * self.weights[label]
prob[label] = math.exp(feat_weight)
Z += prob[label]
for label in self.labels:
prob[label] /= Z
return prob
```
使用方法:
```python
me = MaxEnt()
me.load_data('train.txt')
me.train()
```
其中,`train.txt` 是训练数据集文件,每一行代表一个训练样本,第一个字段是标签,后面是特征。你需要根据你的数据集进行相应的调整。
相关推荐
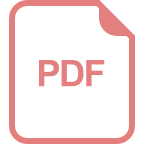
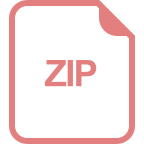
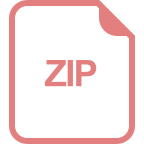














