spring boot 管理员权限登录 给出代码
时间: 2023-07-29 11:13:24 浏览: 87
以下是一个简单的示例代码,演示了如何在 Spring Boot 中实现管理员权限登录:
1. 创建一个 User 实体类
```java
@Entity
@Table(name = "users")
public class User implements Serializable {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false, unique = true)
private String username;
@Column(nullable = false)
private String password;
@Column(nullable = false)
private boolean isAdmin;
// 省略 getter 和 setter 方法
}
```
2. 创建一个 UserRepository 接口
```java
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
```
3. 创建一个 UserDetailsServiceImpl 实现 UserDetailsService 接口
```java
@Service
public class UserDetailsServiceImpl implements UserDetailsService {
@Autowired
private UserRepository userRepository;
@Override
public UserDetails loadUserByUsername(String username) {
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found");
}
List<GrantedAuthority> authorities = new ArrayList<>();
authorities.add(new SimpleGrantedAuthority(user.isAdmin() ? "ADMIN" : "USER"));
return new org.springframework.security.core.userdetails.User(user.getUsername(), user.getPassword(), authorities);
}
}
```
4. 创建一个 SecurityConfig 类
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.defaultSuccessUrl("/")
.permitAll()
.and()
.logout()
.permitAll();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService)
.passwordEncoder(passwordEncoder());
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
5. 创建一个 LoginController 类
```java
@Controller
public class LoginController {
@GetMapping("/login")
public String login() {
return "login";
}
}
```
6. 创建一个 login.html 页面
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Login</title>
</head>
<body>
<h1>Login</h1>
<form action="/login" method="post">
<div>
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
</div>
<button type="submit">Login</button>
</form>
</body>
</html>
```
7. 创建一个 AdminController 类
```java
@Controller
@RequestMapping("/admin")
public class AdminController {
@GetMapping
public String index() {
return "admin/index";
}
}
```
8. 创建一个 admin/index.html 页面
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Admin</title>
</head>
<body>
<h1>Admin Area</h1>
<p>Welcome, admin!</p>
</body>
</html>
```
以上是一个简单的示例代码,演示了如何在 Spring Boot 中实现管理员权限登录。
阅读全文
相关推荐
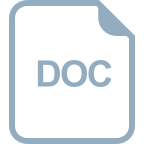
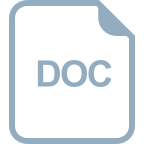
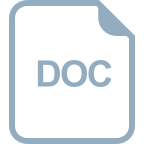
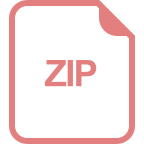
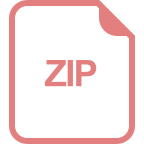
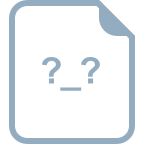
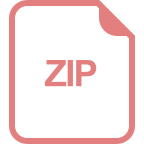
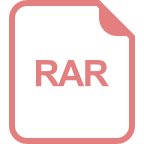
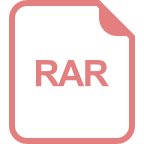
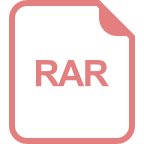
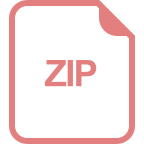
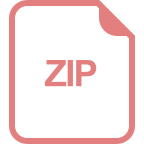
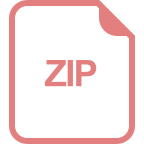
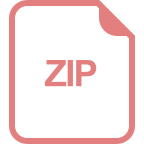
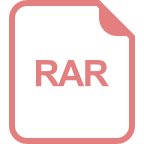
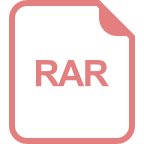
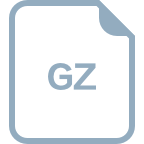
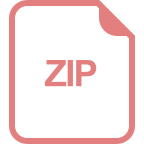
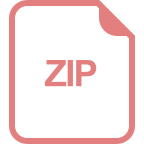