Python报错input X contains NAN
时间: 2024-05-14 21:19:09 浏览: 293
这个错误通常发生在你的数据中存在空值(NaN)。你可以使用以下代码来检测数据中是否存在空值:
```python
import numpy as np
# 生成一个包含空值的数组
a = np.array([1, 2, np.nan, 4])
# 检查数组中是否存在空值
print(np.isnan(a))
```
如果输出结果中有 True 表示存在空值。你需要对数据进行清洗,例如可以使用 pandas 库的 dropna() 方法删除空值:
```python
import pandas as pd
# 读取包含空值的数据
df = pd.read_csv('data.csv')
# 删除包含空值的行
df.dropna(inplace=True)
```
如果你希望将空值替换为其他值,可以使用 fillna() 方法进行填充:
```python
# 将空值替换为 0
df.fillna(0, inplace=True)
```
相关问题
python报错Input contains NaN, infinity or a value too large for dtype('float32').
这个错误通常是由于数据中包含 NaN (Not a Number)、无穷大或太大的值所导致的。你需要先找出数据中的这些异常值,并进行处理。
一种常见的处理方式是将这些异常值替换成一个合适的值,比如平均值、中位数或者其他预先确定的值。你可以使用 pandas 库中的 fillna() 方法来进行替换。
另外,如果你使用的是 TensorFlow 或者 PyTorch 等深度学习框架,你也可以使用 tf.debugging.check_numerics() 或者 torch.isnan() 方法来检查是否存在 NaN 值,并在出现异常时抛出异常。这样可以帮助你更快地定位问题所在。
举个例子,如果你正在处理一个名为 data 的 pandas DataFrame,你可以使用以下代码来替换其中的 NaN 值:
```python
data.fillna(data.mean(), inplace=True)
```
如果你使用的是 TensorFlow,你可以在模型训练前加入以下代码来检查数据是否包含 NaN 值:
```python
import tensorflow as tf
# 检查数据是否包含 NaN 值
tf.debugging.check_numerics(data, "Data contains NaN or Inf")
```
如果你使用的是 PyTorch,你可以在模型训练前加入以下代码来检查数据是否包含 NaN 值:
```python
import torch
# 检查数据是否包含 NaN 值
if torch.isnan(data).any():
raise ValueError("Data contains NaN or Inf")
```
Input X contains NaN.
"NaN" stands for "Not a Number" and is usually encountered in mathematical operations where the result is undefined or cannot be represented as a numerical value.
To handle this error in code, you can use conditional statements to check for NaN before performing any calculations. For example, in Python, you can use the `math.isnan()` function to check if a value is NaN:
```
import math
x = float(input("Enter a number: "))
if math.isnan(x):
print("Input contains NaN")
else:
# perform calculations
```
Alternatively, you can use try-except blocks to catch any exceptions that may arise from NaN values:
```
x = float(input("Enter a number: "))
try:
# perform calculations
except ValueError:
print("Input contains NaN")
```
阅读全文
相关推荐
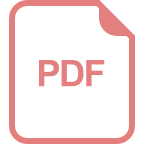
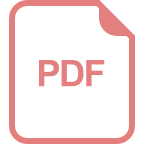













