pandas读取多个excel文件,代码
时间: 2023-09-24 09:11:02 浏览: 68
你可以使用`pandas`库中的`read_excel()`函数来读取多个Excel文件,具体代码如下:
```python
import pandas as pd
import glob
# 获取所有要读取的Excel文件路径
excel_files = glob.glob('path/to/files/*.xlsx')
# 循环读取每个Excel文件,并将它们合并成一个数据框
dfs = []
for file in excel_files:
df = pd.read_excel(file)
dfs.append(df)
merged_df = pd.concat(dfs, ignore_index=True)
```
在上面的代码中,`glob.glob()`函数用于获取指定路径下的所有Excel文件路径。`pd.read_excel()`函数用于读取每个Excel文件,并将它们存储在一个数据框列表中。最后,`pd.concat()`函数用于将这些数据框合并成一个数据框。`ignore_index=True`参数用于重新设置合并后的数据框的索引。
相关问题
pandas可以读取多个excel文件吗
是的,pandas可以读取多个Excel文件。你可以使用Python的os模块来获取文件夹中的所有Excel文件,然后使用pandas中的read_excel()函数逐个读取。以下是一个示例代码:
```python
import os
import pandas as pd
path = '/path/to/folder/containing/excel/files'
files = os.listdir(path)
excels = [file for file in files if file.endswith('.xlsx')]
dfs = []
for excel in excels:
df = pd.read_excel(os.path.join(path, excel))
dfs.append(df)
# 合并数据
result = pd.concat(dfs)
```
在这个例子中,我们首先使用os.listdir()函数获取文件夹中的所有文件,然后使用列表推导式将列表筛选为以.xlsx结尾的Excel文件。接下来,我们使用for循环逐个读取Excel文件,并将它们存储在一个列表中。最后,我们使用pd.concat()函数将所有DataFrame合并为一个DataFrame。
pandas读取两个excel文件并连接查询
### 回答1:
您可以使用Pandas的`read_excel`方法读取两个Excel文件,然后使用`merge`或`concat`方法将它们连接起来。
示例代码:
```
import pandas as pd
df1 = pd.read_excel("file1.xlsx")
df2 = pd.read_excel("file2.xlsx")
# 使用concat方法将两个数据帧连接起来,默认沿着列的方向
result = pd.concat([df1, df2])
# 使用merge方法将两个数据帧按某个特定的列连接起来
result = pd.merge(df1, df2, on='column_name')
```
您也可以使用多种方法对连接后的数据进行筛选和操作,例如使用`groupby`、`pivot_table`等。
### 回答2:
使用pandas可以轻松地读取和处理多个Excel文件,并进行连接查询。
首先,我们需要导入pandas库:
```python
import pandas as pd
```
然后,可以使用`pd.read_excel()`函数加载两个Excel文件,并将它们保存为不同的DataFrame对象:
```python
df1 = pd.read_excel('文件1.xlsx')
df2 = pd.read_excel('文件2.xlsx')
```
接下来,我们可以使用pandas的连接操作将这两个DataFrame对象连接起来。如果两个DataFrame对象具有相同的列名,我们可以使用`pd.concat()`函数进行纵向连接:
```python
df_combined = pd.concat([df1, df2])
```
如果两个DataFrame对象具有相同的行索引,我们可以使用`pd.merge()`函数进行横向连接。首先,我们需要找到用于连接的共同列,并使用`pd.merge()`指定连接方式(inner、outer、left或right):
```python
df_combined = pd.merge(df1, df2, on='共同列名', how='连接方式')
```
完成连接后,我们就可以对合并后的DataFrame进行查询了。可以使用`df_combined[条件]`来选择满足特定条件的行,条件可以是列的某个值的比较、布尔运算等等:
```python
result = df_combined[df_combined['某列名'] > 100]
```
最后,我们可以将查询结果保存为新的Excel文件:
```python
result.to_excel('查询结果.xlsx', index=False)
```
综上所述,通过使用pandas库,我们可以轻松地读取和连接多个Excel文件,并进行各种查询和操作。
### 回答3:
使用pandas可以方便地读取和操作Excel文件,并且可以将两个Excel文件进行连接和查询。
首先,我们需要导入pandas库并读取两个Excel文件。可以使用`pd.read_excel()`函数读取Excel文件并将其转换为pandas的DataFrame对象。假设我们有两个Excel文件分别为 "file1.xlsx" 和 "file2.xlsx",我们可以按照以下方式读取:
```python
import pandas as pd
excel1 = pd.read_excel('file1.xlsx')
excel2 = pd.read_excel('file2.xlsx')
```
接下来,我们可以使用pandas的连接函数`pd.concat()`将两个Excel文件进行连接。连接可以按照行或列的方式进行。如果我们想按照行的方式将两个Excel文件连接起来,可以使用以下代码:
```python
combined = pd.concat([excel1, excel2], axis=0)
```
上述代码将把excel1和excel2按行连接起来形成一个新的DataFrame对象combined。
如果我们想按照列的方式连接两个Excel文件,可以使用以下代码:
```python
combined = pd.concat([excel1, excel2], axis=1)
```
上述代码将把excel1和excel2按列连接起来形成一个新的DataFrame对象combined。
最后,我们可以使用pandas的查询功能对连接之后的DataFrame对象进行查询。可以使用`df[df['column_name'] == value]`进行基于某个列的值进行查询,或者使用`df[df['column_name'].str.contains('value')]`进行模糊查询。
以上是使用pandas读取两个Excel文件并连接查询的基本步骤,具体的查询操作可以根据实际需求进行进一步扩展。
相关推荐
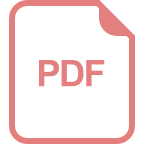
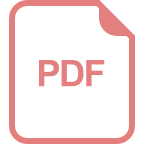
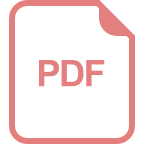












