3. 编程使用消息队列从父进程发送10个整数到子进程,子进程收下来后计算总和,后用同一个消息队列把总和发回给父进程显示。可用整个数组的方式发送,或者用循环逐个元素发送。设计思路
时间: 2024-06-06 08:07:34 浏览: 20
1. 创建消息队列,获取消息队列ID。
2. 创建子进程。
3. 在父进程中,使用消息队列发送10个整数到子进程。
4. 在子进程中,使用消息队列接收10个整数,并计算它们的总和。
5. 在子进程中,使用消息队列将总和发送回父进程。
6. 在父进程中,使用消息队列接收总和,并显示结果。
7. 关闭消息队列。
相关问题
c语言编程使用消息队列从父进程发送10个整数到子进程,子进程收下来后计算总和,后用同一个消息队列把总和发回给父进程显示用循环逐个元素发送
以下是一个简单的实现:
父进程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/msg.h>
#include <sys/ipc.h>
#define MSG_SIZE 1024
struct msgbuf {
long mtype;
int mtext[10];
};
int main() {
int msgid;
key_t key = 1234;
struct msgbuf message;
int sum = 0;
// 创建消息队列
msgid = msgget(key, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
// 发送消息
for (int i = 0; i < 10; i++) {
message.mtype = 1;
message.mtext[i] = i + 1;
if (msgsnd(msgid, &message, sizeof(message.mtext), 0) == -1) {
perror("msgsnd");
exit(EXIT_FAILURE);
}
}
// 接收消息
if (msgrcv(msgid, &message, sizeof(message.mtext), 2, 0) == -1) {
perror("msgrcv");
exit(EXIT_FAILURE);
}
for (int i = 0; i < 10; i++) {
sum += message.mtext[i];
}
printf("Sum: %d\n", sum);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS);
}
```
子进程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/msg.h>
#include <sys/ipc.h>
#define MSG_SIZE 1024
struct msgbuf {
long mtype;
int mtext[10];
};
int main() {
int msgid;
key_t key = 1234;
struct msgbuf message;
int sum = 0;
// 获取消息队列
msgid = msgget(key, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
// 接收消息
if (msgrcv(msgid, &message, sizeof(message.mtext), 1, 0) == -1) {
perror("msgrcv");
exit(EXIT_FAILURE);
}
for (int i = 0; i < 10; i++) {
sum += message.mtext[i];
}
// 发送消息
message.mtype = 2;
for (int i = 0; i < 10; i++) {
message.mtext[i] = 0;
}
message.mtext[0] = sum;
if (msgsnd(msgid, &message, sizeof(message.mtext), 0) == -1) {
perror("msgsnd");
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS);
}
```
子进程和父进程不是同一个rq吗
子进程和父进程在刚刚创建时,在CPU调度器中的调度队列是相同的。也就是说,它们会被放在同一个调度队列中,等待CPU调度器进行调度。这是因为在Linux中,子进程是由父进程通过fork()系统调用创建的,所以它们最初会共享父进程的调度队列。
然而,当子进程开始执行时,它可能会被移动到其他的调度队列中。这是因为在CFS调度算法中,每个调度队列都与一个权重值和运行时间限制相关联。当一个进程在一个调度队列中运行了一段时间后,它的权重值会被调整,以便其他进程也有机会获得CPU时间片。如果一个进程的权重值变得很低,它可能会被移动到另一个调度队列中,以便更高优先级的进程可以获得更多的CPU时间片。因此,子进程和父进程可能会在不同的调度队列中运行。
相关推荐
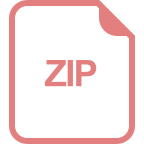
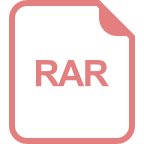
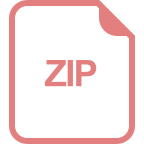
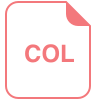












