获取两个DateTime间的所有日期字符串代码
时间: 2023-09-05 10:05:16 浏览: 60
### 回答1:
可以使用以下代码:
from datetime import date, timedelta
def daterange(start_date, end_date):
for n in range(int ((end_date - start_date).days)):
yield start_date + timedelta(n)
start_date = date(2020, 1, 1)
end_date = date(2020, 1, 15)
for single_date in daterange(start_date, end_date):
print(single_date.strftime("%Y-%m-%d"))
### 回答2:
以下是一个获取两个DateTime间的所有日期字符串的示例代码:
```csharp
using System;
using System.Collections.Generic;
public class Program
{
public static void Main(string[] args)
{
DateTime startDate = new DateTime(2022, 1, 1);
DateTime endDate = new DateTime(2022, 1, 10);
List<string> dateStrings = GetDateStrings(startDate, endDate);
foreach(string dateString in dateStrings)
{
Console.WriteLine(dateString);
}
}
public static List<string> GetDateStrings(DateTime startDate, DateTime endDate)
{
List<string> dateStrings = new List<string>();
DateTime currentDate = startDate;
while(currentDate <= endDate) {
dateStrings.Add(currentDate.ToString("yyyy-MM-dd"));
currentDate = currentDate.AddDays(1);
}
return dateStrings;
}
}
```
在上述代码中,我们定义了一个`GetDateStrings`方法,该方法接受两个`DateTime`对象作为参数,并返回一个`List<string>`,其中包含了这两个日期之间的所有日期字符串。
在`Main`方法中,我们使用示例值初始化了`startDate`和`endDate`。然后,我们调用`GetDateStrings`方法,并将返回的日期字符串列表保存在`dateStrings`变量中。
最后,我们使用`foreach`循环遍历`dateStrings`列表,并逐行打印每个日期字符串。
以上代码输出的结果将会是:
```
2022-01-01
2022-01-02
2022-01-03
2022-01-04
2022-01-05
2022-01-06
2022-01-07
2022-01-08
2022-01-09
2022-01-10
```
### 回答3:
获取两个`DateTime`间的所有日期字符串的代码可以使用以下方法:
```csharp
DateTime startDate = DateTime.Parse("2022-01-01");
DateTime endDate = DateTime.Parse("2022-01-10");
List<string> dateStrings = new List<string>();
DateTime currentDate = startDate;
while (currentDate <= endDate)
{
dateStrings.Add(currentDate.ToString("yyyy-MM-dd"));
currentDate = currentDate.AddDays(1);
}
foreach (string dateString in dateStrings)
{
Console.WriteLine(dateString);
}
```
以上代码假设我们有两个`DateTime`对象,`startDate`表示开始日期,`endDate`表示结束日期。我们创建一个空的字符串列表`dateStrings`用于存储所有的日期字符串。
然后我们使用一个`while`循环,将`currentDate`初始化为开始日期。在循环中,我们将`currentDate`的字符串表示(用"yyyy-MM-dd"格式化)添加到`dateStrings`列表中,并将`currentDate`增加一天。循环会一直运行,直到`currentDate`超过结束日期。
最后,我们使用`foreach`循环遍历`dateStrings`列表,将每个日期字符串打印出来。
通过以上代码,我们可以获取到`startDate`和`endDate`之间所有的日期字符串。在这个例子中,输出会是:
```
2022-01-01
2022-01-02
2022-01-03
2022-01-04
2022-01-05
2022-01-06
2022-01-07
2022-01-08
2022-01-09
2022-01-10
```
这些字符串表示了从2022年1月1日到2022年1月10日的所有日期。
相关推荐
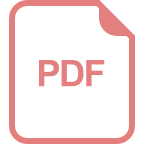















