ofstream 写入文件
时间: 2023-07-26 11:33:36 浏览: 237
ofstream 是C++标准库中的一个输出文件流类,用于向文件中写入数据。其使用方法如下:
1. 包含头文件
```c++
#include <fstream>
```
2. 创建 ofstream 对象
```c++
ofstream ofs;
```
3. 打开文件
```c++
ofs.open("filename.txt");
```
4. 写入数据
```c++
ofs << "Hello, World!";
```
5. 关闭文件
```c++
ofs.close();
```
完整示例代码如下:
```c++
#include <fstream>
#include <iostream>
using namespace std;
int main() {
ofstream ofs;
ofs.open("output.txt");
if (!ofs) {
cerr << "Failed to open file!" << endl;
return 1;
}
ofs << "Hello, World!" << endl;
ofs << "This is a test." << endl;
ofs.close();
return 0;
}
```
以上代码会向名为 output.txt 的文件中写入两行数据。注意,如果打开文件失败,需要及时进行错误处理,避免因为文件操作失败造成不必要的问题。
相关问题
ofstream 写入文件不覆盖
在使用 ofstream 写入文件时,如果不想覆盖原有文件内容,可以使用以下方法:
1. 使用 ios::app 模式打开文件,即在文件末尾追加内容。
```cpp
#include <fstream>
using namespace std;
int main()
{
ofstream outfile("example.txt", ios::app);
outfile << "This is some additional text." << endl;
outfile.close();
return 0;
}
```
2. 使用 ios::in 模式打开文件,即在文件原有内容上继续写入。
```cpp
#include <fstream>
using namespace std;
int main()
{
ofstream outfile("example.txt", ios::in);
outfile.seekp(0, ios::end);
outfile << "This is some additional text." << endl;
outfile.close();
return 0;
}
```
其中,outfile.seekp(0, ios::end) 表示将文件指针移动到文件末尾。
ofstream写入图片
使用 ofstream 写入图片需要先将图片以二进制方式读入内存,然后再将内存中的数据写入到文件中。
以下是一个简单的示例代码:
```c++
#include <fstream>
#include <iostream>
int main() {
// 以二进制方式读取图片
std::ifstream input("example.jpg", std::ios::binary);
if (!input) {
std::cerr << "Failed to open file for reading!\n";
return 1;
}
// 获取文件长度
input.seekg(0, std::ios::end);
std::size_t size = input.tellg();
// 读取文件内容到内存
char* buffer = new char[size];
input.seekg(0, std::ios::beg);
input.read(buffer, size);
input.close();
// 写入文件
std::ofstream output("example_copy.jpg", std::ios::binary);
if (!output) {
std::cerr << "Failed to open file for writing!\n";
return 1;
}
output.write(buffer, size);
output.close();
delete[] buffer;
std::cout << "Done!\n";
return 0;
}
```
该示例代码中,我们先使用 `std::ifstream` 以二进制方式打开要写入的图片文件。接着,我们使用 `seekg` 和 `tellg` 获取文件长度,并将文件内容读取到内存中。最后,我们使用 `std::ofstream` 将内存中的数据写入到新的文件中。
阅读全文
相关推荐
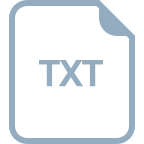












