窗口排队管理系统c++
时间: 2023-08-03 07:22:57 浏览: 33
窗口排队管理系统是一个比较典型的模拟系统,可以用C++来实现。下面是一个简单的实现思路:
1. 定义一个窗口类,包含窗口编号、服务类型等属性,以及服务开始和结束时间的方法。
2. 定义一个顾客类,包含顾客编号、到达时间、等待时间、服务时间等属性,以及等待和服务的方法。
3. 定义一个队列类,用于存储等待服务的顾客。
4. 在主函数中,首先输入窗口数量和服务类型,然后不断输入顾客到达时间和服务时间,将顾客按到达时间排序后加入队列中。
5. 进入循环,每次循环取出一个窗口和一个顾客,让窗口服务该顾客,将该顾客的等待时间计入总等待时间统计中。
6. 直到所有顾客都被服务完毕,输出平均等待时间即可。
代码实现可以参考以下示例:
```c++
#include <iostream>
#include <queue>
using namespace std;
// 窗口类
class Window {
public:
int id; // 窗口编号
int serveTime; // 服务时间
int startTime; // 服务开始时间
int endTime; // 服务结束时间
int serveType; // 服务类型
bool available; // 是否空闲
// 构造函数
Window(int id, int serveType) {
this->id = id;
this->serveType = serveType;
this->available = true;
}
// 开始服务
void startServe(int curTime) {
this->startTime = curTime;
this->endTime = curTime + this->serveTime;
this->available = false;
}
// 结束服务
void endServe() {
this->available = true;
}
};
// 顾客类
class Customer {
public:
int id; // 顾客编号
int arriveTime; // 到达时间
int waitTime; // 等待时间
int serveTime; // 服务时间
// 构造函数
Customer(int id, int arriveTime, int serveTime) {
this->id = id;
this->arriveTime = arriveTime;
this->serveTime = serveTime;
this->waitTime = 0;
}
// 等待服务
void waitServe(int curTime) {
this->waitTime = curTime - this->arriveTime;
}
};
// 队列类
class Queue {
public:
queue<Customer*> customers; // 存储等待的顾客
// 添加顾客
void addCustomer(Customer* customer) {
this->customers.push(customer);
}
// 取出顾客
Customer* getCustomer() {
if (this->customers.empty()) {
return NULL;
}
Customer* customer = this->customers.front();
this->customers.pop();
return customer;
}
};
int main() {
int n, serveType;
cout << "请输入窗口数量和服务类型(1:普通,2:快速,3:VIP): ";
cin >> n >> serveType;
// 初始化窗口
Window* windows[n];
for (int i = 0; i < n; i++) {
windows[i] = new Window(i + 1, serveType);
}
// 输入顾客信息
Queue queue;
int id = 1, arriveTime, serveTime;
while (true) {
cout << "请输入第" << id << "位顾客的到达时间和服务时间(输入-1结束输入): ";
cin >> arriveTime;
if (arriveTime == -1) {
break;
}
cin >> serveTime;
Customer* customer = new Customer(id, arriveTime, serveTime);
queue.addCustomer(customer);
id++;
}
// 开始服务
int curTime = 0, totalWaitTime = 0, servedCount = 0;
while (true) {
// 遍历窗口,找到空闲窗口和等待时间最短的顾客
Window* availableWindow = NULL;
Customer* shortestWaitCustomer = NULL;
int shortestWaitTime = INT_MAX;
for (int i = 0; i < n; i++) {
if (windows[i]->available) {
Customer* customer = queue.getCustomer();
if (customer != NULL && customer->arriveTime <= curTime) {
customer->waitServe(curTime);
if (customer->waitTime < shortestWaitTime) {
shortestWaitTime = customer->waitTime;
shortestWaitCustomer = customer;
availableWindow = windows[i];
}
} else {
windows[i]->endServe();
}
} else {
if (windows[i]->endTime == curTime) {
windows[i]->endServe();
servedCount++;
totalWaitTime += windows[i]->startTime - shortestWaitCustomer->arriveTime;
}
}
}
// 所有顾客都已服务完毕
if (servedCount == id - 1) {
break;
}
// 空闲窗口和等待时间最短的顾客都找到了
if (availableWindow != NULL && shortestWaitCustomer != NULL) {
availableWindow->serveTime = shortestWaitCustomer->serveTime;
availableWindow->startServe(curTime);
}
curTime++;
}
// 输出平均等待时间
double avgWaitTime = (double) totalWaitTime / (id - 1);
cout << "平均等待时间为: " << avgWaitTime << endl;
return 0;
}
```
相关推荐
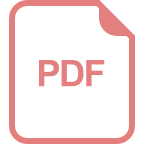
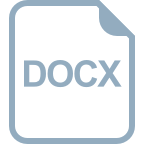
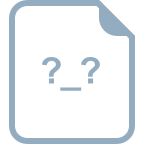











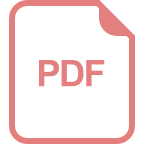
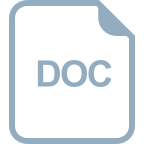
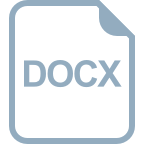
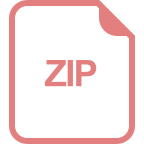