STM32用标准库把GNRMC格式转换成经纬度的代码
时间: 2024-02-27 08:57:10 浏览: 131
假设你已经成功地从GPS模块中读取到了GNRMC格式的定位数据,并将其存储在一个字符串中。那么,以下是一个使用标准库将GNRMC格式转换成经纬度的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#define PI 3.14159265359
typedef struct {
double latitude;
double longitude;
} GPSPosition;
GPSPosition getGPSPosition(char* nmea) {
GPSPosition pos = {0, 0};
char* token;
int i = 0;
char* args[13];
token = strtok(nmea, ",");
while (token != NULL) {
args[i++] = token;
token = strtok(NULL, ",");
}
if (i != 13 || strcmp(args[0], "$GNGGA") != 0) {
printf("Invalid NMEA sentence\n");
return pos;
}
double latitude_degrees = atof(args[2]) / 100;
double latitude_minutes = fmod(atof(args[2]), 100) / 60;
pos.latitude = latitude_degrees + latitude_minutes;
if (args[3][0] == 'S') {
pos.latitude = -pos.latitude;
}
double longitude_degrees = atof(args[4]) / 100;
double longitude_minutes = fmod(atof(args[4]), 100) / 60;
pos.longitude = longitude_degrees + longitude_minutes;
if (args[5][0] == 'W') {
pos.longitude = -pos.longitude;
}
return pos;
}
int main() {
char nmea[] = "$GNGGA,123519,4807.038,N,01131.000,E,1,08,0.9,545.4,M,46.9,M,,*47";
GPSPosition pos = getGPSPosition(nmea);
printf("latitude: %lf\n", pos.latitude);
printf("longitude: %lf\n", pos.longitude);
return 0;
}
```
这段代码将字符串 `"$GNGGA,123519,4807.038,N,01131.000,E,1,08,0.9,545.4,M,46.9,M,,*47"` 作为输入参数传递给 `getGPSPosition()` 函数,并返回一个包含经纬度信息的 `GPSPosition` 结构体。在本例中,该函数首先将输入字符串解析成一个字符串数组,并确保该数组符合GNRMC格式要求。然后,它将纬度和经度从字符串中提取出来,并将它们转换成浮点数。最后,它根据北纬/南纬和东经/西经的标识符确定对应的符号,并返回一个 `GPSPosition` 结构体。
请注意,此代码仅适用于GNRMC格式的数据,如果输入的字符串不符合该格式,该函数将返回一个经纬度都为0的结构体。此外,此代码还假设您已经将从GPS模块中读取到的数据存储在一个字符串中。在实际应用中,您需要编写代码来从串口或其他接口读取数据。
阅读全文
相关推荐
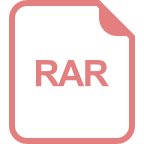



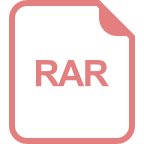
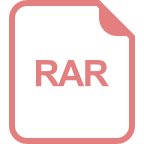
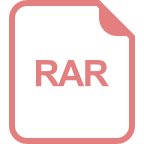
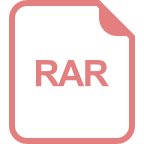
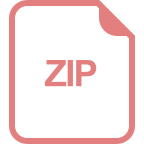
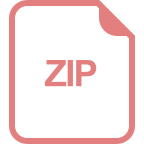
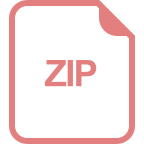
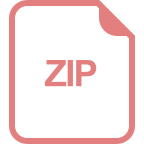
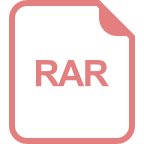
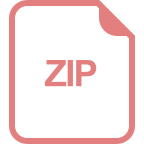
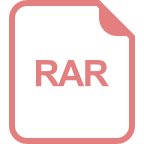
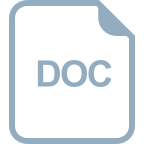