nuscenes 点云 框 显示
时间: 2023-09-23 18:06:45 浏览: 218
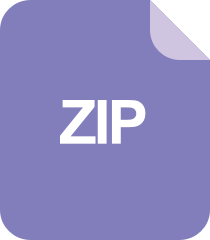
点云打开与显示

您可以使用nuscenes库中的代码来显示nuscenes点云框。以下是一些示例代码:
1. 在nuscenes可视化工具中显示点云框
```python
from nuscenes.nuscenes import NuScenes
from nuscenes.utils.data_classes import LidarPointCloud
from nuscenes.utils.geometry_utils import BoxVisibility, transform_matrix
nusc = NuScenes(version='v1.0-mini', dataroot='/data/nuscenes', verbose=True)
# Get a lidar point cloud and transform it to the ego vehicle frame.
sample_rec = nusc.sample[0]
lidar_token = sample_rec['data']['LIDAR_TOP']
lidar_data = nusc.get('sample_data', lidar_token)
pc = LidarPointCloud.from_file(nusc.get_sample_data_path(lidar_token))
ego_pose = nusc.get('ego_pose', lidar_data['ego_pose_token'])
calib = nusc.get('calibrated_sensor', lidar_data['calibrated_sensor_token'])
lidar_points = pc.points[:3, :] # Take the first 3 rows because the fourth row is reflectance.
# Transform the point cloud to the ego vehicle frame.
lidar_points = transform_matrix(ego_pose['translation'], ego_pose['rotation'], inverse=False) @ lidar_points
lidar_points = transform_matrix(calib['translation'], calib['rotation'], inverse=False) @ lidar_points
# Get the annotations for the first sample.
annotations = nusc.get('sample_annotation', sample_rec['anns'])
# Set up the visualization.
vis = nuscenes.utils.visualizations.NuscenesVisualizer(nusc=nusc)
# Loop over the annotations and add them to the visualization.
for i, ann in enumerate(annotations):
# Only show boxes that are visible from the lidar point cloud.
if not BoxVisibility.isVisible(ann['bbox'], lidar_points):
continue
# Get the box corners and transform them to the ego vehicle frame.
corners = nuscenes.utils.geometry_utils.get_3d_box(ann, nusc)
corners = transform_matrix(ego_pose['translation'], ego_pose['rotation'], inverse=False) @ corners
corners = transform_matrix(calib['translation'], calib['rotation'], inverse=False) @ corners
# Add the box to the visualization.
vis.add_box(corners, label=ann['category_name'], color='red', alpha=0.5)
# Render the visualization.
vis.render()
```
2. 使用matplotlib库显示点云框
```python
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d.art3d import Poly3DCollection
# Get the annotations for the first sample.
annotations = nusc.get('sample_annotation', sample_rec['anns'])
# Set up the plot.
fig = plt.figure(figsize=(10, 10))
ax = fig.add_subplot(111, projection='3d')
ax.set_xlim3d(-50, 50)
ax.set_ylim3d(-50, 50)
ax.set_zlim3d(-5, 5)
# Loop over the annotations and add them to the plot.
for i, ann in enumerate(annotations):
# Only show boxes that are visible from the lidar point cloud.
if not BoxVisibility.isVisible(ann['bbox'], lidar_points):
continue
# Get the box corners and transform them to the ego vehicle frame.
corners = nuscenes.utils.geometry_utils.get_3d_box(ann, nusc)
corners = transform_matrix(ego_pose['translation'], ego_pose['rotation'], inverse=False) @ corners
corners = transform_matrix(calib['translation'], calib['rotation'], inverse=False) @ corners
# Add the box to the plot.
facecolor = 'red'
edgecolor = 'black'
alpha = 0.5
collection = Poly3DCollection([corners.T[:4], corners.T[4:8], [corners.T[0], corners.T[4], corners.T[5], corners.T[1]], [corners.T[1], corners.T[5], corners.T[6], corners.T[2]], [corners.T[2], corners.T[6], corners.T[7], corners.T[3]], [corners.T[3], corners.T[7], corners.T[4], corners.T[0]]], alpha=alpha, facecolor=facecolor, edgecolor=edgecolor)
ax.add_collection(collection)
# Plot the lidar points.
ax.scatter(lidar_points[0], lidar_points[1], lidar_points[2], s=0.1)
# Show the plot.
plt.show()
```
这些示例代码可以帮助您显示nuscenes点云框。
阅读全文
相关推荐





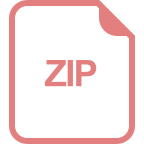



