帮我写一个python代码爬取https://www.amazon.com/SAMSUNG-Factory-Unlocked-Android-Smartphone/product-reviews/B0BLP57HTN/ref=cm_cr_dp_d_show_all_btm?ie=UTF8&reviewerType=all_reviews的评论,并将其分词,清洗后进行情感分析
时间: 2024-04-21 09:30:03 浏览: 231
好的,为了实现这个功能,我们需要使用Python中的一些额外库。具体来说,我们需要使用requests库来发送HTTP请求,使用BeautifulSoup库来解析HTML页面,使用nltk库进行分词和情感分析。
如果你还没有安装nltk库,可以通过以下命令在终端中安装:
```bash
pip install nltk
```
接下来是代码示例:
```python
import requests
from bs4 import BeautifulSoup
from nltk.sentiment.vader import SentimentIntensityAnalyzer
from nltk.tokenize import sent_tokenize, word_tokenize
def analyze_review(review):
sid = SentimentIntensityAnalyzer()
sentences = sent_tokenize(review)
compound_scores = []
for sentence in sentences:
words = word_tokenize(sentence)
compound_scores.append(sid.polarity_scores(sentence)['compound'])
return sum(compound_scores) / len(compound_scores)
def clean_text(text):
# 去除多余空格和换行符
text = text.strip()
# 去除标点符号
text = ''.join(c for c in text if c.isalnum() or c.isspace())
# 转换为小写
text = text.lower()
return text
def get_reviews(url):
# 发送请求
response = requests.get(url)
# 解析HTML页面
soup = BeautifulSoup(response.text, 'html.parser')
# 找到评论区的div
reviews = soup.find_all('div', {'class': 'a-section review aok-relative'})
# 遍历每个评论并提取信息
for review in reviews:
# 提取评论的标题
title = review.find('a', {'class': 'a-size-base a-link-normal review-title a-color-base review-title-content a-text-bold'}).text.strip()
# 提取评论的内容
content = review.find('span', {'class': 'a-size-base review-text review-text-content'}).text.strip()
# 清洗评论文本
content = clean_text(content)
# 进行情感分析
score = analyze_review(content)
# 输出评论信息和情感分数
print(f'Title: {title}\nContent: {content}\nScore: {score}\n\n')
# 爬取的商品评论页面URL
url = 'https://www.amazon.com/SAMSUNG-Factory-Unlocked-Android-Smartphone/product-reviews/B0BLP57HTN/ref=cm_cr_dp_d_show_all_btm?ie=UTF8&reviewerType=all_reviews'
get_reviews(url)
```
这个代码示例会爬取指定亚马逊商品的评论,并对每个评论进行情感分析。具体来说,它会使用NLTK中的VADER情感分析器对每个句子进行情感分析,然后将所有句子的分数取平均值作为整个评论的情感分数。请注意,情感分析并不是一项完美的技术,它的准确性和可信度都受到很多因素的影响,因此结果仅供参考。
阅读全文
相关推荐
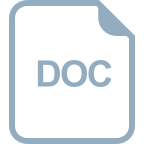
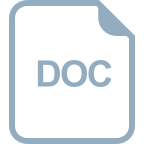
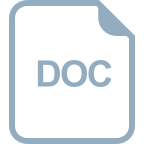


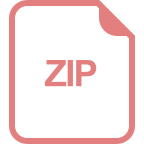




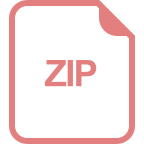
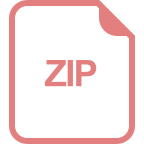





