基于Java为我设计实现一个打怪升级小游戏
时间: 2023-05-23 13:05:43 浏览: 180
A:好的,以下是一个基于Java实现的打怪升级小游戏示例:
首先,我们需要设计游戏中所需的角色和怪物类,以及它们的属性和方法。假设我们的游戏中有玩家角色和怪物角色,它们都有姓名、等级、血量、攻击力和防御力属性。
```
// 角色类
public abstract class Character {
String name;
int level;
int health;
int attack;
int defense;
public abstract void attack(Character target);
public abstract void levelUp()
}
// 玩家类
public class Player extends Character {
int experience;
public Player(String name, int level, int health, int attack, int defense) {
this.name = name;
this.level = level;
this.health = health;
this.attack = attack;
this.defense = defense;
this.experience = 0;
}
public void attack(Character target) {
int damage = this.attack - target.defense;
target.health -= damage;
System.out.println(this.name + "攻击了" + target.name + ", 造成了" + damage + "点伤害!");
if (target.health <= 0) {
System.out.println(target.name + "已经不能战斗了!");
this.experience += target.level * 10;
levelUp();
}
}
public void levelUp() {
if (this.experience >= this.level * 100) {
this.experience -= this.level * 100;
this.level++;
System.out.println(this.name + "升级了,当前等级为" + this.level + "!")
this.health += 10;
this.attack += 5;
this.defense += 5;
}
}
}
// 怪物类
public class Monster extends Character {
public Monster(String name, int level, int health, int attack, int defense) {
this.name = name;
this.level = level;
this.health = health;
this.attack = attack;
this.defense = defense;
}
public void attack(Character target) {
int damage = this.attack - target.defense;
target.health -= damage;
System.out.println(this.name + "攻击了" + target.name + ", 造成了" + damage + "点伤害!");
if (target.health <= 0) {
System.out.println(target.name + "已经不能战斗了!");
}
}
}
```
接着,我们设计游戏管理类,负责控制游戏的流程和事件。在游戏初始化时,随机生成若干只怪物,并让玩家与它们进行战斗。当玩家击败所有怪物后,玩家等级提升,再次与新的怪物战斗,直到达到最高等级或失败。
```
//游戏管理类
public class GameEngine {
List<Character> enemies;
Player player;
public GameEngine(String playerName) {
List<Integer> levels = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> healths = Arrays.asList(10, 20, 30, 40, 50);
List<Integer> attacks = Arrays.asList(5, 10, 15, 20, 25);
List<Integer> defenses = Arrays.asList(5, 10, 15, 20, 25);
Random random = new Random();
enemies = new ArrayList<Character>();
for (int i = 0; i < 5; i++) {
Monster monster = new Monster("怪物" + (i+1), levels.get(random.nextInt(levels.size())), healths.get(random.nextInt(healths.size())), attacks.get(random.nextInt(attacks.size())), defenses.get(random.nextInt(defenses.size())));
enemies.add(monster);
}
player = new Player(playerName, 1, 50, 20, 10);
}
public void start() {
System.out.println("游戏开始!");
for (Character enemy : enemies) {
while (player.health > 0 && enemy.health > 0) {
player.attack(enemy);
if (enemy.health <= 0) {
break;
}
enemy.attack(player);
}
}
System.out.println("当前等级为" + player.level + ", 经验值为" + player.experience + ", 准备下一场战斗!");
while (player.health > 0 && player.level < 5) {
Monster monster = new Monster("怪物" + (player.level + 1), player.level + 1, 50 + player.level * 10, 20 + player.level * 5, 10 + player.level * 5);
System.out.println("出现新的怪物:" + monster.name + "!");
while (player.health > 0 && monster.health > 0) {
player.attack(monster);
if (monster.health <= 0) {
break;
}
monster.attack(player);
}
}
if (player.health <= 0) {
System.out.println(player.name + "被击败了,游戏结束!");
} else {
System.out.println("恭喜" + player.name + "升到了最高等级" + player.level + ",游戏结束!");
}
}
}
```
最后,在主函数中创建游戏管理对象并启动游戏:
```
public static void main(String[] args) {
String playerName = "玩家1";
GameEngine game = new GameEngine(playerName);
game.start();
}
```
以上是一个简单的打怪升级小游戏示例,您可以根据自己的需求和想法添加更多的游戏元素和玩法。
阅读全文
相关推荐
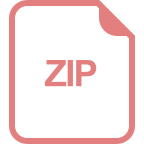
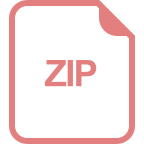
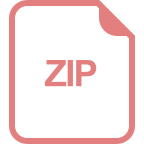















