请用java语言,写一个java对mysql数据库的连接读写示例代码
时间: 2024-03-14 15:45:22 浏览: 34
好的,以下是一个基本的Java连接MySQL数据库并进行读写操作的示例代码:
```java
import java.sql.*;
public class MySQLConn {
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 1. 加载MySQL的JDBC驱动程序
Class.forName("com.mysql.jdbc.Driver");
// 2. 获取MySQL数据库连接
String url = "jdbc:mysql://localhost:3306/testdb"; // testdb为数据库名称
String user = "root"; // 数据库用户名
String password = "123456"; // 数据库密码
conn = DriverManager.getConnection(url, user, password);
// 3. 创建Statement对象,用于执行SQL语句
stmt = conn.createStatement();
// 4. 执行SQL语句,读取数据
String sql = "SELECT * FROM student";
rs = stmt.executeQuery(sql);
while (rs.next()) {
String name = rs.getString("name");
int age = rs.getInt("age");
System.out.println("Name: " + name + ", Age: " + age);
}
// 5. 执行SQL语句,写入数据
sql = "INSERT INTO student(name, age) VALUES('Tom', 20)";
int result = stmt.executeUpdate(sql);
if (result > 0) {
System.out.println("数据写入成功!");
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 6. 释放资源
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
以上代码中,我们首先通过 `Class.forName()` 方法加载MySQL的JDBC驱动程序,然后使用 `DriverManager.getConnection()` 方法获取MySQL数据库连接。接着,我们创建 `Statement` 对象,用于执行SQL语句。通过 `stmt.executeQuery()` 和 `stmt.executeUpdate()` 方法分别执行读和写操作。最后,我们在 `finally` 块中释放资源。
相关推荐
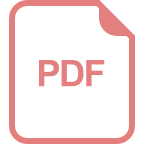
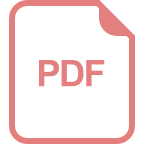
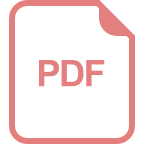
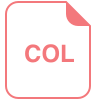
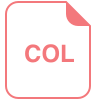
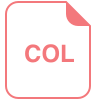
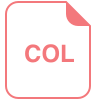
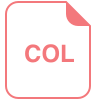









