怎么使用Windows SDK和DirectX SDK来构建dxgi截屏
时间: 2024-04-29 18:21:19 浏览: 125
以下是使用Windows SDK和DirectX SDK来构建dxgi截屏的步骤:
1. 安装Windows SDK和DirectX SDK
2. 创建工程,并将以下头文件包含进来:
```
#include <d3d11.h>
#include <dxgi1_2.h>
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
```
3. 创建一个D3D11设备和设备上下文:
```
D3D_FEATURE_LEVEL featureLevel = D3D_FEATURE_LEVEL_11_0;
D3D11CreateDevice(NULL, D3D_DRIVER_TYPE_HARDWARE, NULL, 0, &featureLevel, 1, D3D11_SDK_VERSION, &m_pd3dDevice, NULL, &m_pd3dDeviceContext);
```
4. 获取DXGI设备:
```
IDXGIDevice* pDXGIDevice;
m_pd3dDevice->QueryInterface(__uuidof(IDXGIDevice), (void**)&pDXGIDevice);
```
5. 获取DXGI适配器:
```
IDXGIAdapter* pDXGIAdapter;
pDXGIDevice->GetParent(__uuidof(IDXGIAdapter), (void**)&pDXGIAdapter);
```
6. 获取DXGI工厂:
```
IDXGIFactory* pIDXGIFactory;
pDXGIAdapter->GetParent(__uuidof(IDXGIFactory), (void**)&pIDXGIFactory);
```
7. 枚举所有显示器:
```
std::vector<IDXGIOutput*> arrOutputs;
IDXGIOutput* pOutput;
for (int i = 0; pIDXGIFactory->EnumOutputs(i, &pOutput) != DXGI_ERROR_NOT_FOUND; ++i) {
arrOutputs.push_back(pOutput);
}
```
8. 获取输出的描述:
```
DXGI_OUTPUT_DESC outputDesc;
arrOutputs[0]->GetDesc(&outputDesc);
```
9. 获取DXGI输出:
```
IDXGIOutput1* pDXGIOutput1;
arrOutputs[0]->QueryInterface(__uuidof(IDXGIOutput1), (void**)&pDXGIOutput1);
```
10. 获取桌面信息:
```
DXGI_OUTDUPL_DESC outputDuplDesc;
pDXGIOutput1->DuplicateOutput(m_pd3dDevice, &outputDuplDesc);
```
11. 创建DXGI资源:
```
ID3D11Texture2D* pTexture;
D3D11_TEXTURE2D_DESC desc;
desc.Width = outputDuplDesc.ModeDesc.Width;
desc.Height = outputDuplDesc.ModeDesc.Height;
desc.Format = outputDuplDesc.ModeDesc.Format;
desc.ArraySize = 1;
desc.BindFlags = D3D11_BIND_FLAG::D3D11_BIND_RENDER_TARGET;
desc.Usage = D3D11_USAGE::D3D11_USAGE_DEFAULT;
desc.SampleDesc.Count = 1;
desc.SampleDesc.Quality = 0;
desc.MipLevels = 1;
desc.CPUAccessFlags = 0;
desc.MiscFlags = 0;
m_pd3dDevice->CreateTexture2D(&desc, NULL, &pTexture);
```
12. 创建渲染目标视图:
```
ID3D11RenderTargetView* pRenderTargetView;
m_pd3dDevice->CreateRenderTargetView(pTexture, NULL, &pRenderTargetView);
```
13. 设置渲染目标:
```
m_pd3dDeviceContext->OMSetRenderTargets(1, &pRenderTargetView, NULL);
```
14. 渲染:
```
m_pd3dDeviceContext->ClearRenderTargetView(pRenderTargetView, DirectX::Colors::Black);
m_pd3dDeviceContext->Draw(3, 0);
```
15. 保存截屏:
```
D3D11_TEXTURE2D_DESC desc;
pTexture->GetDesc(&desc);
D3D11_MAPPED_SUBRESOURCE mappedResource;
m_pd3dDeviceContext->Map(pTexture, 0, D3D11_MAP_READ, 0, &mappedResource);
std::ofstream ofs("screenshot.bmp", std::ios::binary);
const uint8_t* src = (const uint8_t*)mappedResource.pData;
for (int y = 0; y < desc.Height; ++y) {
const uint8_t* row = src + mappedResource.RowPitch * y;
for (int x = 0; x < desc.Width; ++x) {
const uint8_t* pixel = row + x * 4;
ofs.put(pixel[2]);
ofs.put(pixel[1]);
ofs.put(pixel[0]);
}
}
m_pd3dDeviceContext->Unmap(pTexture, 0);
ofs.close();
```
以上就是使用Windows SDK和DirectX SDK来构建dxgi截屏的步骤。需要注意的是,如果您正在使用Windows 10或更高版本,则可以使用Windows.Graphics.Capture命名空间来实现更简单的截屏。
阅读全文
相关推荐
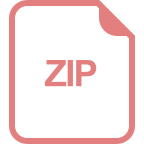
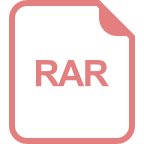
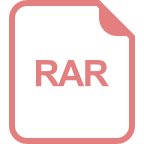















