请写一个带头部尾部的OC瀑布流列表
时间: 2024-02-03 21:12:08 浏览: 29
可以使用UICollectionView来实现一个带头部尾部的OC瀑布流列表,具体步骤如下:
1.创建UICollectionViewFlowLayout对象,设置itemSize、minimumInteritemSpacing、minimumLineSpacing、headerReferenceSize和footerReferenceSize等属性,以实现瀑布流列表效果。
2.创建UICollectionView对象并设置delegate和dataSource。
3.实现UICollectionViewDataSource协议中的方法,包括numberOfSectionsInCollectionView、collectionView:numberOfItemsInSection和collectionView:cellForItemAtIndexPath等方法,以显示列表内容。
4.实现UICollectionViewDelegateFlowLayout协议中的方法,包括collectionView:layout:sizeForItemAtIndexPath、collectionView:layout:referenceSizeForHeaderInSection和collectionView:layout:referenceSizeForFooterInSection等方法,以设置item和头部尾部视图的大小。
5.在需要显示头部尾部视图的地方,通过UICollectionReusableView来创建头部尾部视图,并返回给collectionView。
6.最后,在UICollectionViewFlowLayout的属性中设置sectionHeadersPinToVisibleBounds和sectionFootersPinToVisibleBounds为YES,以实现头部尾部视图的悬停效果。
下面是一个简单的示例代码:
```
// 创建 UICollectionViewFlowLayout 对象
UICollectionViewFlowLayout *flowLayout = [[UICollectionViewFlowLayout alloc] init];
flowLayout.itemSize = CGSizeMake(itemWidth, itemHeight);
flowLayout.minimumInteritemSpacing = 10;
flowLayout.minimumLineSpacing = 10;
flowLayout.headerReferenceSize = CGSizeMake(0, headerHeight);
flowLayout.footerReferenceSize = CGSizeMake(0, footerHeight);
// 创建 UICollectionView 对象
UICollectionView *collectionView = [[UICollectionView alloc] initWithFrame:self.view.bounds collectionViewLayout:flowLayout];
collectionView.delegate = self;
collectionView.dataSource = self;
collectionView.backgroundColor = [UIColor whiteColor];
[self.view addSubview:collectionView];
// 实现 UICollectionViewDataSource 协议方法
- (NSInteger)numberOfSectionsInCollectionView:(UICollectionView *)collectionView {
return sectionCount;
}
- (NSInteger)collectionView:(UICollectionView *)collectionView numberOfItemsInSection:(NSInteger)section {
return itemCounts[section];
}
- (UICollectionViewCell *)collectionView:(UICollectionView *)collectionView cellForItemAtIndexPath:(NSIndexPath *)indexPath {
MyCollectionViewCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:@"MyCell" forIndexPath:indexPath];
// 设置 cell 的内容
return cell;
}
// 实现 UICollectionViewDelegateFlowLayout 协议方法
- (CGSize)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout *)collectionViewLayout sizeForItemAtIndexPath:(NSIndexPath *)indexPath {
return CGSizeMake(itemWidth, itemHeight);
}
- (CGSize)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout *)collectionViewLayout referenceSizeForHeaderInSection:(NSInteger)section {
return CGSizeMake(0, headerHeight);
}
- (CGSize)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout *)collectionViewLayout referenceSizeForFooterInSection:(NSInteger)section {
return CGSizeMake(0, footerHeight);
}
// 创建头部尾部视图
- (UICollectionReusableView *)collectionView:(UICollectionView *)collectionView viewForSupplementaryElementOfKind:(NSString *)kind atIndexPath:(NSIndexPath *)indexPath {
if ([kind isEqualToString:UICollectionElementKindSectionHeader]) {
MyHeaderView *headerView = [collectionView dequeueReusableSupplementaryViewOfKind:UICollectionElementKindSectionHeader withReuseIdentifier:@"MyHeader" forIndexPath:indexPath];
// 设置 headerView 的内容
return headerView;
} else if ([kind isEqualToString:UICollectionElementKindSectionFooter]) {
MyFooterView *footerView = [collectionView dequeueReusableSupplementaryViewOfKind:UICollectionElementKindSectionFooter withReuseIdentifier:@"MyFooter" forIndexPath:indexPath];
// 设置 footerView 的内容
return footerView;
}
return nil;
}
// 悬停头部尾部视图
- (void)collectionView:(UICollectionView *)collectionView willDisplaySupplementaryView:(UICollectionReusableView *)view forElementKind:(NSString *)elementKind atIndexPath:(NSIndexPath *)indexPath {
UICollectionViewFlowLayout *layout = (UICollectionViewFlowLayout *)collectionView.collectionViewLayout;
if ([elementKind isEqualToString:UICollectionElementKindSectionHeader]) {
layout.sectionHeadersPinToVisibleBounds = YES;
} else if ([elementKind isEqualToString:UICollectionElementKindSectionFooter]) {
layout.sectionFootersPinToVisibleBounds = YES;
}
}
```
以上代码仅供参考,实际使用时需要根据自己的需求进行相应的修改和优化。
相关推荐
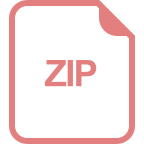
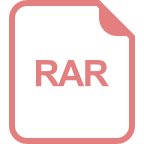
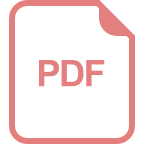














