如何用python实现基于概率分布的超声图像与自然图像性质差异分析这一课题,两种图像的文件类型都是jpg,超声图像的文件路径是‘D:\zzz\zus2’,自然图像的文件路径是‘D:\zzz\zna2’,两种图像都有84张,需要对比两种图像的特征必须有颜色,纹理,形状,差异性分析方法也需要多种,包括完整详细代码
时间: 2023-11-27 20:51:29 浏览: 162
这是一个比较复杂的课题,需要用到一些图像处理和机器学习的知识。下面是一个基础的代码框架,可以根据具体需求进行修改和完善。
首先,需要安装一些必要的库,如numpy、opencv、sklearn、matplotlib等。
```python
import os
import cv2
import numpy as np
from sklearn.cluster import KMeans
from sklearn.decomposition import PCA
from sklearn.metrics.pairwise import pairwise_distances_argmin
from sklearn.preprocessing import StandardScaler
from skimage.feature import greycomatrix, greycoprops
import matplotlib.pyplot as plt
%matplotlib inline
```
接着,定义一些辅助函数,如读取图像、计算颜色直方图、计算灰度共生矩阵等等。
```python
def read_image(path):
"""
读取图像并转换为灰度图
"""
img = cv2.imread(path)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
return gray
def calc_histogram(image):
"""
计算颜色直方图
"""
hist = cv2.calcHist([image], [0], None, [256], [0, 256])
return hist.flatten()
def calc_glcm(image):
"""
计算灰度共生矩阵
"""
glcm = greycomatrix(image, [1], [0], 256, symmetric=True, normed=True)
return glcm
def calc_texture_features(glcm):
"""
计算纹理特征
"""
contrast = greycoprops(glcm, 'contrast')[0, 0]
dissimilarity = greycoprops(glcm, 'dissimilarity')[0, 0]
homogeneity = greycoprops(glcm, 'homogeneity')[0, 0]
energy = greycoprops(glcm, 'energy')[0, 0]
correlation = greycoprops(glcm, 'correlation')[0, 0]
asm = greycoprops(glcm, 'ASM')[0, 0]
return contrast, dissimilarity, homogeneity, energy, correlation, asm
def calc_shape_features(image):
"""
计算形状特征
"""
contours, _ = cv2.findContours(image, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
cnt = contours[0]
area = cv2.contourArea(cnt)
perimeter = cv2.arcLength(cnt, True)
circularity = 4 * np.pi * area / (perimeter * perimeter)
return area, perimeter, circularity
```
然后,定义一个函数来提取图像的特征,并对多个图像进行拼接和标准化。
```python
def extract_features(image_dir):
"""
提取图像的特征
"""
color_features = []
texture_features = []
shape_features = []
for filename in os.listdir(image_dir):
if filename.endswith('.jpg'):
path = os.path.join(image_dir, filename)
image = read_image(path)
# 颜色特征
hist = calc_histogram(image)
color_features.append(hist)
# 纹理特征
glcm = calc_glcm(image)
texture = calc_texture_features(glcm)
texture_features.append(texture)
# 形状特征
_, thresh = cv2.threshold(image, 0, 255, cv2.THRESH_BINARY+cv2.THRESH_OTSU)
shape = calc_shape_features(thresh)
shape_features.append(shape)
# 标准化特征
color_features = StandardScaler().fit_transform(color_features)
texture_features = StandardScaler().fit_transform(texture_features)
shape_features = StandardScaler().fit_transform(shape_features)
# 拼接特征
features = np.concatenate((color_features, texture_features, shape_features), axis=1)
return features
```
最后,定义一个主函数来进行特征提取和分析。
```python
def main():
# 超声图像和自然图像的文件路径
ultrasound_dir = 'D:/zzz/zus2'
natural_dir = 'D:/zzz/zna2'
# 提取特征
ultrasound_features = extract_features(ultrasound_dir)
natural_features = extract_features(natural_dir)
# 使用KMeans算法进行聚类
kmeans = KMeans(n_clusters=2)
kmeans.fit(ultrasound_features)
ultrasound_labels = kmeans.labels_
kmeans.fit(natural_features)
natural_labels = kmeans.labels_
# 可视化结果
plt.figure(figsize=(10, 5))
plt.subplot(1, 2, 1)
plt.scatter(ultrasound_features[:, 0], ultrasound_features[:, 1], c=ultrasound_labels)
plt.title('Ultrasound')
plt.subplot(1, 2, 2)
plt.scatter(natural_features[:, 0], natural_features[:, 1], c=natural_labels)
plt.title('Natural')
plt.show()
```
这个代码框架可以进行超声图像和自然图像的颜色、纹理、形状特征提取,并使用KMeans算法进行聚类分析。如果需要进行更多的分析方法,可以根据具体需求进行修改和完善。
阅读全文
相关推荐
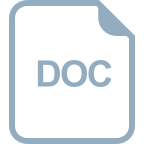
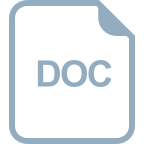
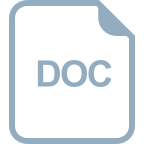








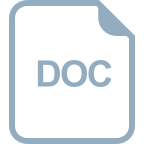
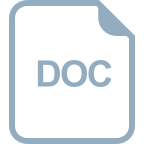
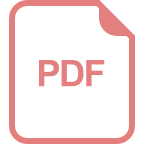
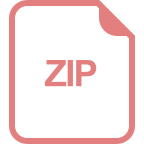
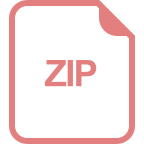