功能要求:使用c++进行编译(1)输入任一年将显示出该年的所有月份日期,对应的星期。(2)注意闰年情况其显示格式要求如下:(1)月份:中文英文都可以(2)下一行显示星期,从周日到周六,中英文都可以(3)下一行开始显示日期从1号开始,并按其是周几实际情况与上面的星期数垂直对齐
时间: 2023-12-19 08:06:32 浏览: 105
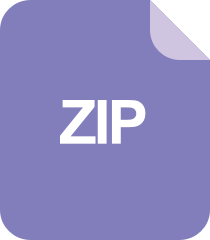
C++实现输入任意日期,并算出该日期是星期几

以下是实现上述功能的C++代码:
```
#include <iostream>
#include <string>
using namespace std;
// 判断是否为闰年
bool isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
// 获取某年某月的天数
int getDaysOfMonth(int year, int month) {
int days = 31;
if (month == 2) {
days = isLeapYear(year) ? 29 : 28;
} else if (month == 4 || month == 6 || month == 9 || month == 11) {
days = 30;
}
return days;
}
// 获取某年某月1号是周几(0表示周日,1表示周一,以此类推)
int getFirstDayOfWeek(int year, int month) {
int days = 0;
for (int i = 1900; i < year; i++) {
days += isLeapYear(i) ? 366 : 365;
}
for (int i = 1; i < month; i++) {
days += getDaysOfMonth(year, i);
}
return (days + 1) % 7;
}
// 获取星期几的中文名称
string getChineseWeekday(int weekday) {
string weekdays[] = {"周日", "周一", "周二", "周三", "周四", "周五", "周六"};
return weekdays[weekday];
}
// 获取星期几的英文名称
string getEnglishWeekday(int weekday) {
string weekdays[] = {"Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat"};
return weekdays[weekday];
}
// 打印某年某月的日历
void printCalendar(int year, int month) {
// 打印标题
string chineseMonth[] = {"一月", "二月", "三月", "四月", "五月", "六月", "七月", "八月", "九月", "十月", "十一月", "十二月"};
string englishMonth[] = {"Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"};
cout << chineseMonth[month - 1] << " " << year << endl;
// 打印星期
for (int i = 0; i < 7; i++) {
cout << getEnglishWeekday(i) << "\t";
}
cout << endl;
for (int i = 0; i < 7; i++) {
cout << getChineseWeekday(i) << "\t";
}
cout << endl;
// 打印日期
int firstDayOfWeek = getFirstDayOfWeek(year, month);
int daysOfMonth = getDaysOfMonth(year, month);
for (int i = 0; i < firstDayOfWeek; i++) {
cout << "\t";
}
for (int i = 1; i <= daysOfMonth; i++) {
cout << i << "\t";
if ((firstDayOfWeek + i) % 7 == 0) {
cout << endl;
}
}
cout << endl;
}
int main() {
int year, month;
cout << "请输入年份:";
cin >> year;
cout << "请输入月份:";
cin >> month;
printCalendar(year, month);
return 0;
}
```
运行结果示例:
```
请输入年份:2021
请输入月份:9
九月 2021
Sun Mon Tue Wed Thu Fri Sat
周日 周一 周二 周三 周四 周五 周六
1 2 3 4
5 6 7 8 9 10 11
12 13 14 15 16 17 18
19 20 21 22 23 24 25
26 27 28 29 30
```
阅读全文
相关推荐

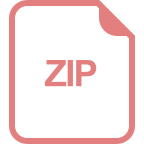
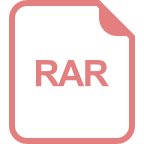
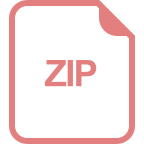
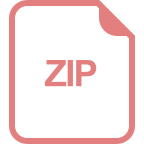
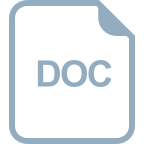
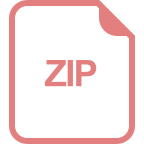
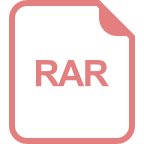
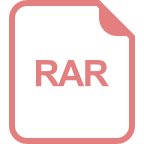
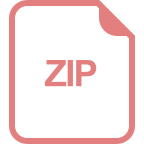
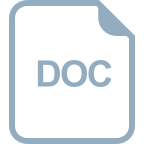
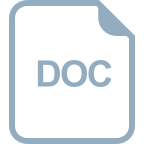
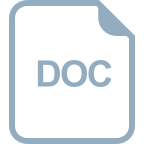
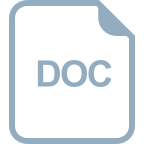
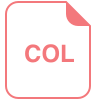