Java ParameterizedTypeReference
时间: 2024-03-09 14:39:52 浏览: 190
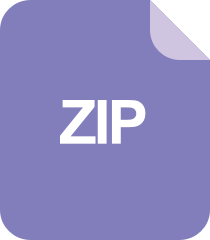
Java教程练习.zip
Java ParameterizedTypeReference is a class that is used to represent a generic type at runtime. It is a useful class when dealing with generic types, especially when working with RESTful APIs that return data in JSON format.
When working with RESTful APIs, the response data is usually returned in JSON format. The JSON data is then deserialized into Java objects using a library such as Jackson. However, when dealing with generic types such as a List of objects, it is not possible to deserialize the JSON data into the correct type without additional information about the type.
This is where the ParameterizedTypeReference class comes in. It provides a way to represent a generic type at runtime, which can then be used by Jackson to correctly deserialize the JSON data.
Here is an example of using ParameterizedTypeReference with Jackson to deserialize a JSON array into a List of objects:
```
RestTemplate restTemplate = new RestTemplate();
ResponseEntity<List<MyObject>> responseEntity = restTemplate.exchange(
"http://api.example.com/my-objects",
HttpMethod.GET,
null,
new ParameterizedTypeReference<List<MyObject>>() {}
);
List<MyObject> myObjects = responseEntity.getBody();
```
In this example, we use the RestTemplate class to make a GET request to the API endpoint that returns a JSON array of MyObject objects. We pass a ParameterizedTypeReference object to the restTemplate.exchange() method, which provides information about the generic type we want to deserialize the JSON data into.
The ParameterizedTypeReference class is defined as an anonymous inner class, which is necessary because Java's type erasure means that generic type information is not available at runtime. The {} at the end of the class definition is an empty initialization block, which is necessary to create an instance of the anonymous inner class.
The result of the API call is stored in a ResponseEntity object, which contains the response headers and body. We can then extract the List of MyObject objects from the response body using the getBody() method.
Overall, the ParameterizedTypeReference class is a useful tool when working with generic types in Java, especially when dealing with RESTful APIs that return data in JSON format.
阅读全文
相关推荐
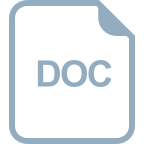
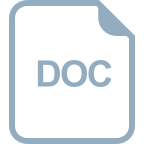

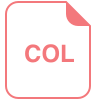
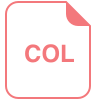
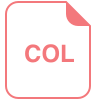











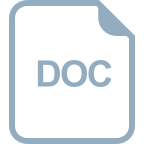