Java微服务架构与Spring Cloud实践
发布时间: 2024-03-27 07:57:28 阅读量: 13 订阅数: 17 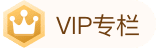
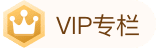
# 1. 微服务架构概述
- 1.1 什么是微服务架构?
- 1.2 微服务架构优势与挑战
- 1.3 微服务架构与传统单体架构对比
# 2. Spring Cloud简介
- 2.1 Spring Cloud概述
- 2.2 Spring Cloud的核心组件
- 2.3 Spring Cloud与微服务架构的关系
# 3. 服务注册与发现
### 3.1 服务注册与发现的基本概念
在微服务架构中,服务注册与发现是非常重要的一环。简单地说,服务注册是指微服务将自己的信息(比如IP地址、端口号、服务名称等)注册到一个服务注册中心,而服务发现则是指微服务通过查询服务注册中心来发现其它服务的位置信息。
### 3.2 Spring Cloud Eureka实践
Spring Cloud提供了Eureka作为服务注册中心,通过Eureka可以很方便地实现微服务的注册与发现。以下是一个简单的示例代码:
```java
// 服务提供者
@SpringBootApplication
@EnableEurekaClient
@RestController
public class ProductServiceApplication {
@GetMapping("/products/{id}")
public String getProductById(@PathVariable long id) {
return "Product Id: " + id;
}
public static void main(String[] args) {
SpringApplication.run(ProductServiceApplication.class, args);
}
}
// 服务消费者
@SpringBootApplication
@EnableEurekaClient
@RestController
public class ProductClientApplication {
@Autowired
private RestTemplate restTemplate;
@GetMapping("/products/{id}")
public String getProduct(@PathVariable long id) {
return restTemplate.getForObject("http://product-service/products/" + id, String.class);
}
public static void main(String[] args) {
SpringApplication.run(ProductClientApplication.class, args);
}
}
// 应用配置
spring.application.name=product-service
server.port=8081
eureka.client.serviceUrl.defaultZone=http://localhost:8761/eureka
eureka.instance.hostname=localhost
eureka.client.regiset-with-eureka=true
eureka.client.fetch-registry=true
```
### 3.3 服务注册中心的高可用性设计
为了保证服务注册中心的高可用性,通常会采用集群部署的方式,将多个Eureka实例组成一个集群。在Spring Cloud中,只需修改配置指定多个Eureka服务地址即可实现高可用性,例如:
```yaml
eureka:
client:
serviceUrl:
defaultZone: http://eureka1:8761/eureka,http://eureka2:8762/eureka
```
通过以上配置,可以将服务注册中心扩展为两个实例,保证了在一个实例出现故障时,系统仍能正常运行。
# 4. 服务间通信
### 4.1 RESTful API设计原则
在微服务架构中,服务间通信是至关重要的。而RESTful API作为一种通用的设计风格,被广泛应用于微服务的通信中。以下是一些常见的RESTful API设计原则:
1. **资源路径**:使用名词表示资源,遵循RESTful风格的URL路径设计,如`/users`、`/orders`。
2. **HTTP方法**:使用HTTP动词对资源进行操作,GET(查询)、POST(创建)、PUT(更新)、DELETE(删除)等。
3. **状态码**:合理利用HTTP状态码,如200表示成功、404表示资源未找到、500表示服务器错误等。
4. **版本控制**:为API添加版本号,如`/v1/users`、`/v2/users`,以便进行版本管理。
5. **参数传递**:使用查询参数进行过滤、分页等操作,如`/users?page=1&size=10`。
6. **错误处理**:统一格式返回错误信息,包括错误码、错误描述等,便于客户端处理异常情况。
### 4.2 Spring Cloud Feign实现服务调用
Spring Cloud Feign是一个基于Netflix Feign的声明式、模板化的
0
0
相关推荐
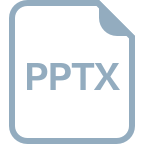
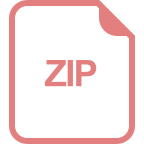





