C# 在三维坐标系中 写一个过三点的二次贝塞尔曲线 详细代码
时间: 2024-03-28 07:42:23 浏览: 128
以下是 C# 中过三点的二次贝塞尔曲线的代码:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
public partial class Form1 : Form
{
private Point[] points = new Point[3];
private Pen curvePen = new Pen(Color.Blue, 2);
private Pen pointPen = new Pen(Color.Red, 5);
public Form1()
{
InitializeComponent();
this.DoubleBuffered = true; // 双缓冲
}
private void Form1_MouseClick(object sender, MouseEventArgs e)
{
if (e.Button == MouseButtons.Left)
{
for (int i = 0; i < 3; i++)
{
if (points[i].IsEmpty)
{
points[i] = e.Location;
break;
}
}
if (!points[2].IsEmpty)
{
this.Invalidate(); // 重绘
}
}
}
private void Form1_Paint(object sender, PaintEventArgs e)
{
if (!points[2].IsEmpty)
{
// 计算贝塞尔曲线上的所有点
Point[] curvePoints = new Point[101];
for (int i = 0; i <= 100; i++)
{
float t = i / 100f;
float x = (1 - t) * (1 - t) * points[0].X + 2 * t * (1 - t) * points[1].X + t * t * points[2].X;
float y = (1 - t) * (1 - t) * points[0].Y + 2 * t * (1 - t) * points[1].Y + t * t * points[2].Y;
curvePoints[i] = new Point((int)x, (int)y);
}
// 绘制贝塞尔曲线和控制点
e.Graphics.DrawBezier(curvePen, points[0], points[1], points[2], curvePoints[100]);
for (int i = 0; i < 3; i++)
{
e.Graphics.DrawEllipse(pointPen, points[i].X - pointPen.Width / 2, points[i].Y - pointPen.Width / 2, pointPen.Width, pointPen.Width);
}
}
}
}
```
这个代码实现了在一个窗体上通过鼠标点击三个点,然后绘制过这三个点的二次贝塞尔曲线。在 `Form1_Paint` 方法中,我们使用 `DrawBezier` 方法绘制贝塞尔曲线,使用 `DrawEllipse` 方法绘制控制点。在 `Form1_MouseClick` 方法中,我们记录三个点的坐标,并在第三个点被记录时调用 `Invalidate` 方法重绘窗体。
阅读全文
相关推荐
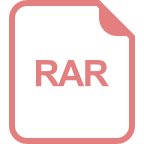
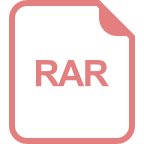
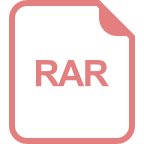
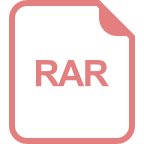
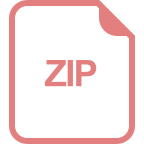
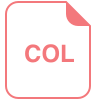
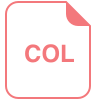
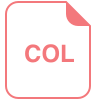
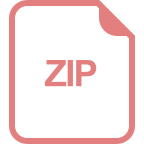