用pytorch写一段预测酒精浓度的代码
时间: 2023-05-11 17:05:11 浏览: 79
以下是使用 PyTorch 编写的预测酒精浓度的代码:
```python
import torch
import torch.nn as nn
# 定义神经网络模型
class AlcoholNet(nn.Module):
def __init__(self):
super(AlcoholNet, self).__init__()
self.fc1 = nn.Linear(10, 5)
self.fc2 = nn.Linear(5, 1)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
# 加载模型
model = AlcoholNet()
model.load_state_dict(torch.load('alcohol_model.pth'))
# 预测函数
def predict_alcohol(features):
with torch.no_grad():
features = torch.tensor(features).float()
output = model(features)
return output.item()
# 使用模型进行预测
features = [0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9, 1.0]
predicted_alcohol = predict_alcohol(features)
print('Predicted alcohol concentration:', predicted_alcohol)
```
请注意,这只是一个示例代码,实际上,要预测酒精浓度,需要使用更多的特征和更复杂的模型。
阅读全文
相关推荐
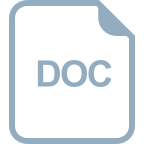
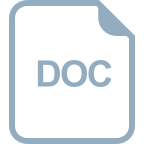
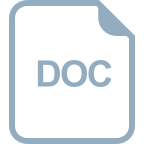
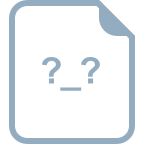
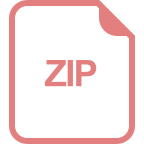
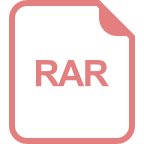
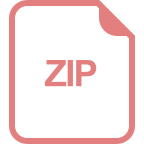
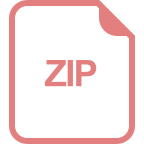
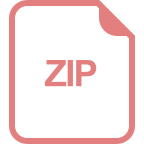
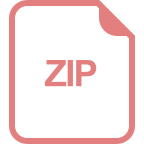
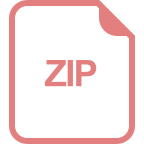
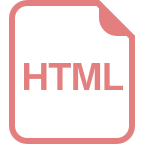
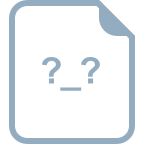