用C语言实现模拟银行大厅叫号功能
时间: 2024-01-21 22:22:37 浏览: 27
好的,我可以为您提供一个简单的模拟银行大厅叫号的实现思路,具体代码实现可以根据您的需求进行修改和完善。
1. 定义一个结构体作为队列元素,包含如下信息:
```c
struct queue_element {
int number; // 排队号码
char name[20]; // 客户姓名
};
```
2. 定义一个队列,并实现基本的队列操作(如队列初始化、入队、出队等),示例代码如下:
```c
#define MAX_QUEUE_SIZE 100
struct queue {
struct queue_element data[MAX_QUEUE_SIZE];
int front, rear;
};
void queue_init(struct queue *q)
{
q->front = q->rear = 0;
}
int is_queue_empty(struct queue *q)
{
return q->front == q->rear;
}
int is_queue_full(struct queue *q)
{
return (q->rear + 1) % MAX_QUEUE_SIZE == q->front;
}
void enqueue(struct queue *q, struct queue_element e)
{
if (is_queue_full(q)) {
fprintf(stderr, "queue is full\n");
exit(1);
}
q->data[q->rear] = e;
q->rear = (q->rear + 1) % MAX_QUEUE_SIZE;
}
struct queue_element dequeue(struct queue *q)
{
if (is_queue_empty(q)) {
fprintf(stderr, "queue is empty\n");
exit(1);
}
struct queue_element e = q->data[q->front];
q->front = (q->front + 1) % MAX_QUEUE_SIZE;
return e;
}
```
3. 定义一个全局变量 `int current_number = 0` 表示当前的排队号码,每次有客户到来时,将其排队号码设为 `++current_number`,并加入队列。示例代码如下:
```c
struct queue queue;
void new_customer(char *name)
{
struct queue_element e;
e.number = ++current_number;
strcpy(e.name, name);
enqueue(&queue, e);
printf("%s 您好,您的排队号码是 %d,请耐心等待\n", e.name, e.number);
}
```
4. 定义一个函数 `call_next`,每次调用该函数时,从队列中取出下一个客户并输出其信息。示例代码如下:
```c
void call_next()
{
if (is_queue_empty(&queue)) {
printf("当前无人排队\n");
return;
}
struct queue_element e = dequeue(&queue);
printf("请 %s 前往 %d 号窗口办理业务\n", e.name, e.number);
}
```
5. 在主函数中循环读入用户命令(如 `new_customer`、`call_next` 等),并调用相应的函数执行操作。示例代码如下:
```c
int main()
{
queue_init(&queue);
while (1) {
char cmd[20], name[20];
printf("> ");
scanf("%s", cmd);
if (strcmp(cmd, "new_customer") == 0) {
scanf("%s", name);
new_customer(name);
} else if (strcmp(cmd, "call_next") == 0) {
call_next();
} else if (strcmp(cmd, "exit") == 0) {
break;
} else {
printf("无效命令,请重新输入\n");
}
}
return 0;
}
```
以上就是一个简单的模拟银行大厅叫号的实现思路,希望能对您有所帮助。
相关推荐
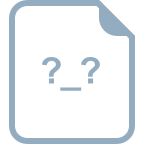














