利用链队列实现银行排队叫号问题
时间: 2023-07-16 08:15:34 浏览: 179
链队列可以用来实现银行排队叫号问题。具体实现步骤如下:
1. 定义一个链队列结构体,包含队头指针和队尾指针。
2. 定义一个叫号函数,每当有客户来到银行,就生成一个新的结点,并将其插入队尾。
3. 定义一个服务函数,每当柜台空闲时,从队头取出一个结点,并将其删除。
4. 定义一个显示队列函数,用于显示当前队列中等待服务的客户的信息。
下面是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int num; // 客户号码
struct Node* next;
} Node;
typedef struct Queue {
Node* front; // 队头指针
Node* rear; // 队尾指针
} Queue;
// 初始化队列
void initQueue(Queue* q) {
q->front = q->rear = NULL;
}
// 判断队列是否为空
int isQueueEmpty(Queue* q) {
return q->front == NULL;
}
// 入队
void enQueue(Queue* q, int num) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->num = num;
newNode->next = NULL;
if (isQueueEmpty(q)) {
q->front = q->rear = newNode;
} else {
q->rear->next = newNode;
q->rear = newNode;
}
}
// 出队
int deQueue(Queue* q) {
if (isQueueEmpty(q)) {
printf("Queue is empty!\n");
return -1;
}
Node* p = q->front;
int num = p->num;
q->front = q->front->next;
free(p);
if (q->front == NULL) {
q->rear = NULL;
}
return num;
}
// 显示队列中的客户号码
void displayQueue(Queue* q) {
Node* p = q->front;
printf("Queue: ");
while (p != NULL) {
printf("%d ", p->num);
p = p->next;
}
printf("\n");
}
int main() {
Queue q;
initQueue(&q);
int choice, num;
do {
printf("1. Generate a new customer number\n");
printf("2. Serve a customer\n");
printf("3. Display the queue\n");
printf("0. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
num = rand() % 1000 + 1; // 生成一个随机的客户号码
enQueue(&q, num);
printf("New customer number: %d\n", num);
break;
case 2:
num = deQueue(&q);
if (num != -1) {
printf("Serving customer number: %d\n", num);
}
break;
case 3:
displayQueue(&q);
break;
case 0:
printf("Exit!\n");
break;
default:
printf("Invalid choice!\n");
break;
}
} while (choice != 0);
return 0;
}
```
这个程序可以通过菜单来模拟银行排队叫号的过程。每当选择 1 时,就会生成一个新的客户号码,并将其插入队尾;每当选择 2 时,就会从队头取出一个客户号码,并将其删除;每当选择 3 时,就会显示当前队列中等待服务的客户的信息。
阅读全文
相关推荐
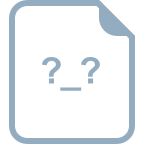





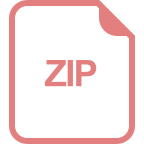
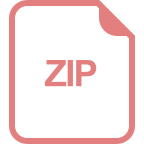
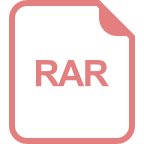
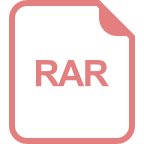
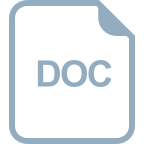
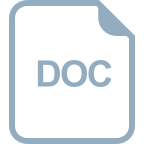




