用STM32F103模拟I2C对HYM8563进行读写,有没有代码例程
时间: 2024-02-10 17:10:40 浏览: 29
是的,以下是使用STM32F103模拟I2C对HYM8563进行读写的代码例程:
```c
#include "stm32f10x.h"
#include "delay.h"
#include "i2c_simulate.h"
#define RTC_ADDR 0xD0
void RTC_Write(uint8_t addr, uint8_t data)
{
// Start Condition
I2C_Start();
I2C_SendByte(RTC_ADDR);
I2C_WaitAck();
// Send Address
I2C_SendByte(addr);
I2C_WaitAck();
// Send data
I2C_SendByte(data);
I2C_WaitAck();
// Stop Condition
I2C_Stop();
}
uint8_t RTC_Read(uint8_t addr)
{
uint8_t data;
// Start Condition
I2C_Start();
I2C_SendByte(RTC_ADDR);
I2C_WaitAck();
// Send Address
I2C_SendByte(addr);
I2C_WaitAck();
// Start Condition
I2C_Start();
I2C_SendByte(RTC_ADDR | 0x01);
I2C_WaitAck();
// Read Data
data = I2C_ReadByte();
I2C_SendAck(1);
// Stop Condition
I2C_Stop();
return data;
}
int main(void)
{
uint8_t data;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
// I2C pins
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6 | GPIO_Pin_7;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_OD;
GPIO_Init(GPIOB, &GPIO_InitStructure);
while (1)
{
// Write to RTC
RTC_Write(0x00, 0x00); // Write 0 to seconds register
// Read from RTC
data = RTC_Read(0x00); // Read seconds register
}
}
```
需要注意的是,这里使用的是软件模拟I2C,因此需要自行实现I2C_Start、I2C_Stop、I2C_SendByte、I2C_ReadByte、I2C_WaitAck和I2C_SendAck函数。其中,I2C_Start函数用于产生起始信号,I2C_Stop函数用于产生停止信号,I2C_SendByte函数用于发送一个字节的数据,I2C_ReadByte函数用于读取一个字节的数据,I2C_WaitAck函数用于等待应答信号,I2C_SendAck函数用于发送应答信号。
相关推荐
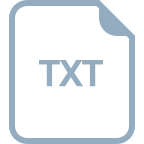














