#include<stdio.h> #include<time.h> #include<stdlib.h> void main() { inta[10],i; srand(time(0)); for(i=0;i<10;i++) a[i]=rand()%90+10;//随机产生一组[10,99]之间的整数 for(i=0;i<10;i++) printf("%d,",a[i]); }
时间: 2024-03-31 09:32:05 浏览: 56
这段代码是用C语言编写的,它的功能是生成一个包含10个随机整数的数组,这些整数的范围是[10,99]。具体实现的步骤是:
1. 引入需要使用的头文件:stdio.h、time.h和stdlib.h。
2. 定义一个包含10个整数的数组a和一个用于循环的变量i。
3. 使用srand函数,根据当前时间设置随机数生成器的种子,以确保每次运行程序时生成的随机数序列都是不同的。
4. 使用for循环生成随机数并存入数组a中。rand函数生成的随机数是[0,RAND_MAX]之间的整数,通过取模运算和加法运算将它们转换为[10,99]之间的整数。
5. 使用for循环遍历数组a,并使用printf函数输出每个元素的值。
需要注意的是,这段代码的main函数没有返回值类型,应该将其改为int类型,并在程序结束时返回0。
相关问题
#include <stdio.h> #include <stdlib.h> #include<sys/types.h> #include <sys/stat.h> #include <fcntl.h> #include <unistd.h> #include <errno.h> #include <stropts.h> #include <time.h> #include <strings.h> #include <string.h> #include <stdio.h> #include <sys/ipc.h> #include <sys/msg.h> struct msg { long msg_types; char msg_buf[511]; }; int main(void) { int qid; int pid; int len; struct msg pmsg; pmsg.msg_types = getpid(); sprintf(pmsg.msg_buf, "hello!this is :%d\n\0", getpid()); len = strlen(pmsg.msg_buf); if ((qid = msgget(IPC_PRIVATE, IPC_CREAT | 0666)) < 0) { perror("msgget"); exit(1); } if ((msgsnd(qid, &pmsg, len, 0)) < 0) { perror("magsn"); exit(1); } printf("successfully send a message to the queue: %d \n", qid); exit(0); }的运行结果
该程序是一个使用消息队列进行进程间通信的示例程序,通过msgget()函数创建一个消息队列,通过msgsnd()函数向消息队列中发送一条消息。
运行结果可能如下:
successfully send a message to the queue: 123456
解释如下:
1.程序首先定义了一个消息结构体msg,并初始化了其中的消息类型msg_types和消息内容msg_buf。
2.程序调用msgget()函数创建一个新的消息队列,将返回的队列ID存储在变量qid中。
3.程序调用msgsnd()函数向消息队列中发送一条消息,消息内容为msg_buf中的字符串。
4.程序输出"successfully send a message to the queue: qid",表示消息发送成功。
需要注意的是,程序中将消息类型msg_types设置为了当前进程的PID,这样在接收消息时可以根据PID来获取对应的消息。同时,程序中使用了strlen()函数来获取消息内容的长度,这是为了确保发送的消息长度不超过队列的最大长度。
#include <iostream> #include <stdio.h> #include <stdlib.h> #include <string.h> using namespace std; class a{ public: int name; int number; void set_name(int ne) { name=ne; } void set_number(int nr) { number=nr; } void show_name() { cout << name; } void show_number() { cout << number; } }; int main() { a a1; a*p; int x; cin>>x; p->set_number(x); p->show_name(); };
在你的代码中,你定义了一个指向类 a 的指针 p,但是你没有为其分配内存空间,因此在使用该指针时会发生未定义行为。你需要使用 new 运算符为该指针分配内存空间,或者直接定义一个类 a 的对象,不使用指针。另外,在 show_name 函数中,你应该输出 name 而不是 number。修改后的代码如下:
```
#include <iostream>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
using namespace std;
class a{
public:
int name;
int number;
void set_name(int ne) {
name = ne;
}
void set_number(int nr) {
number = nr;
}
void show_name() {
cout << name;
}
void show_number() {
cout << number;
}
};
int main() {
a a1;
int x;
cin >> x;
a1.set_number(x);
a1.show_name();
return 0;
}
```
这个程序的功能为:从标准输入中读取一个整数,将其设置为 a1 的 number 值,然后输出 a1 的 name 值。
阅读全文
相关推荐
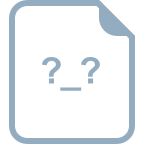
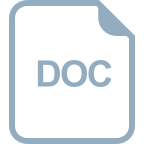
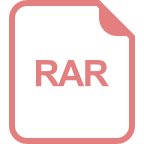












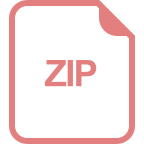
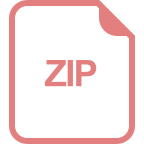
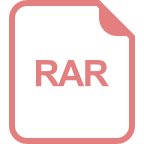