C++中怎么进行四舍五入输出
时间: 2024-05-02 22:21:16 浏览: 71
可以使用标准库函数round()进行四舍五入。round()函数的原型为:
```c
double round(double x);
```
其中,x为要进行四舍五入的浮点数。
使用示例:
```c
#include <stdio.h>
#include <math.h>
int main()
{
double x = 3.45;
double y = 6.78;
printf("%.0f\n", round(x)); // 输出4
printf("%.0f\n", round(y)); // 输出7
return 0;
}
```
注意:round()函数只能对浮点型进行四舍五入,如果要对整型进行四舍五入,可以使用强制类型转换的方法。例如:
```c
int x = 345;
int y = 678;
printf("%d\n", (int)round((double)x/100.0)*100); // 输出300
printf("%d\n", (int)round((double)y/100.0)*100); // 输出700
```
相关问题
C++如何进行四舍五入
在C++中,你可以使用库函数来进行四舍五入操作。主要有几种方法:
1. **std::round()**:这是C++标准库提供的函数,它对整数进行四舍五入。例如:
```cpp
#include <cmath>
int rounded_value = std::round(3.7); // 输出4
```
2. **std::nearbyint()**:这个函数返回给定值的最接近的整数,通常用于浮点数的四舍五入。如果结果等于给定值,则该值将被舍去。例如:
```cpp
int rounded_value = std::nearbyint(3.5f); // 输出3
```
3. **std::trunc(), floor(), ceil()**:这三个函数分别表示截断(去掉小数部分)、向下取整和向上取整。如果你只需要舍去小数,`std::trunc()`可以派上用场:
```cpp
double value = 3.9;
int truncated_value = std::trunc(value); // 输出3
```
4. **自定义函数**:如果需要更复杂的规则,比如银行家的舍入(逢五进一),可以编写自己的函数。
注意:对于浮点数,四舍五入的行为取决于硬件和编译设置,因为它们不是精确的。在处理金钱等敏感数值时,应特别注意这一点。
c++怎么判断四舍五入
在C++中,可以使用函数round()来实现四舍五入操作。round()函数的原型如下:
```c++
double round(double x);
```
该函数会将参数x进行四舍五入操作,并返回最接近x的整数值。如果x与两个整数相同距离相等,则返回偶数值。
例如:
```c++
#include <iostream>
#include <cmath>
int main() {
double x = 3.49;
double y = 3.51;
double z = 3.5;
std::cout << "round(x) = " << round(x) << std::endl; // 输出3
std::cout << "round(y) = " << round(y) << std::endl; // 输出4
std::cout << "round(z) = " << round(z) << std::endl; // 输出4
return 0;
}
```
需要注意的是,round()函数是C++11标准中新增的函数,如果你使用的是较老的编译器,可能会出现编译错误,需要升级编译器或者使用其他方法实现四舍五入操作。
阅读全文
相关推荐
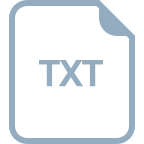













