帮我一个稀疏注意力机制代码,用pytorch架构
时间: 2024-05-08 11:19:22 浏览: 13
当然,我可以帮你写稀疏注意力机制的代码,以下是一个简单的实现:
```
import torch
import torch.nn as nn
import torch.nn.functional as F
class SparseAttention(nn.Module):
def __init__(self, dim, num_heads):
super().__init__()
self.dim = dim
self.num_heads = num_heads
self.q_layer = nn.Linear(dim, dim * num_heads)
self.k_layer = nn.Linear(dim, dim * num_heads)
self.v_layer = nn.Linear(dim, dim * num_heads)
def calc_attention_scores(self, q, k):
scores = torch.matmul(q, k.transpose(-1, -2))
scores *= self.dim ** -0.5
return scores
def forward(self, x):
batch_size, seq_len, dim = x.shape
q = self.q_layer(x).view(batch_size, seq_len, self.num_heads, dim).permute(0, 2, 1, 3)
k = self.k_layer(x).view(batch_size, seq_len, self.num_heads, dim).permute(0, 2, 3, 1)
v = self.v_layer(x).view(batch_size, seq_len, self.num_heads, dim).permute(0, 2, 1, 3)
attn_scores = self.calc_attention_scores(q, k) # (batch_size, num_heads, seq_len, seq_len)
attn_weights = F.softmax(attn_scores, dim=-1)
# Create random sparsity pattern for each sample in batch
patterns = torch.bernoulli(0.5 * torch.ones(batch_size, self.num_heads, seq_len, seq_len))
attn_weights = attn_weights * patterns
out = torch.matmul(attn_weights, v).permute(0, 2, 1, 3).contiguous()
out = out.view(batch_size, seq_len, self.num_heads * dim)
return out
```
这是一个基础实现,你可以根据自己的具体需求进行修改和优化。希望能对你有所帮助!
相关推荐
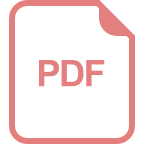
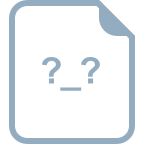
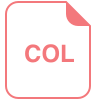














