import numpy as np # b = np.load("train_od_3936_109_109.npy") # print(b) c = np.load("X_od.npy") D = np.load("Y_od.npy") print(c.shape) print(D.shape) max=np.max(c) train_x=c[0:1000]/max train_y=D[0:1000]/max val_x=c[1000:1150]/max val_y=D[1000:1150]/max test_x=c[1150:]/max twst_y=D[1150:] print(train_x.shape) # print(D.shape) print(val_x.shape) # print(D.shape) print(test_x.shape) # print(D.shape) from keras.layers import Dense, LSTM, ConvLSTM2D, Dropout, Reshape from keras.models import Sequential model = Sequential() model.add(Reshape((5,109,109,1),input_shape=(5,109,109))) model.add(ConvLSTM2D(filters=64, kernel_size=(3, 3), activation='relu', padding='same',input_shape=(5, 109, 109, 1))) # model.add(Dropout(0.2)) model.add(Dense(1)) # 在Dense层中,输出维度应该是(109, 109, 1),而不是1 model.add(Reshape((109, 109))) # 在Reshape层中,输出维度应该是(109, 109)而不是(5, 109, 109)。 model.summary() model.compile(optimizer='adam', loss='mse') history = model.fit(train_x, train_y, epochs=50, batch_size=32, validation_data=(val_x, val_y), verbose=1, shuffle=False)该段程序怎么改为以LSTM神经网络模型的地铁客流预测和预测结果可视化代码
时间: 2023-06-26 10:03:17 浏览: 61
import numpy as np
import matplotlib.pyplot as plt
from keras.layers import Dense, LSTM, Dropout, Reshape
from keras.models import Sequential
# 加载数据
c = np.load("X_od.npy")
D = np.load("Y_od.npy")
max_val = np.max(c)
train_x = c[:1000] / max_val
train_y = D[:1000] / max_val
val_x = c[1000:1150] / max_val
val_y = D[1000:1150] / max_val
test_x = c[1150:] / max_val
test_y = D[1150:] / max_val
# LSTM神经网络模型
model = Sequential()
model.add(LSTM(units=64, input_shape=(5, 109), return_sequences=True))
model.add(Dropout(0.2))
model.add(LSTM(units=32))
model.add(Dense(units=109, activation='linear'))
model.summary()
model.compile(optimizer='adam', loss='mse')
# 模型训练
history = model.fit(train_x, train_y, epochs=50, batch_size=32,
validation_data=(val_x, val_y), verbose=1, shuffle=False)
# 模型预测
train_predict = model.predict(train_x)
val_predict = model.predict(val_x)
test_predict = model.predict(test_x)
# 预测结果可视化
plt.figure(figsize=(20, 8))
plt.plot(train_y[-100:], label='true')
plt.plot(train_predict[-100:], label='predict')
plt.legend()
plt.title('Training set')
plt.show()
plt.figure(figsize=(20, 8))
plt.plot(val_y[-50:], label='true')
plt.plot(val_predict[-50:], label='predict')
plt.legend()
plt.title('Validation set')
plt.show()
plt.figure(figsize=(20, 8))
plt.plot(test_y[:50], label='true')
plt.plot(test_predict[:50], label='predict')
plt.legend()
plt.title('Test set')
plt.show()
相关推荐
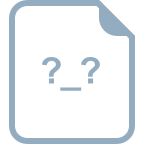
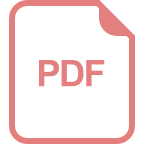
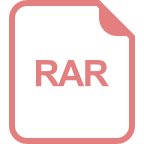















