chunks = torch.chunk(x, 3, dim=0)如何将他们融合
时间: 2023-12-12 16:05:45 浏览: 24
如果想将分块后的张量重新合并成一个张量,可以使用torch.cat()函数。例如,如果要将分成3块的张量`x`在0维度上合并,可以使用以下代码:
```
x = torch.randn(9, 2)
chunks = torch.chunk(x, 3, dim=0)
merged = torch.cat(chunks, dim=0)
```
这里假设`x`是一个9行2列的张量,将它在0维度上分成3块。`torch.chunk()`函数返回一个包含3个分块张量的元组,通过`torch.cat()`函数将这些张量在0维度上合并成一个张量`merged`。
相关问题
优化这段代码让其在gpu上可以更快运行:import torch.nn as nnclass MyLinear(nn.Module): def __init__(self, in_features, out_features): super(MyLinear, self).__init__() self.linears = nn.ModuleList([nn.Linear(in_features, out_features) for i in range(5)]) def forward(self, x): chunks = torch.chunk(x, 5, dim=1) chunks = torch.stack(chunks, dim=0) weights = torch.stack([linear.weight for linear in self.linears], dim=0) outputs = torch.matmul(chunks, weights.transpose(1, 2)).reshape(x.shape[0], -1) return outputs
可以使用 PyTorch 内置的函数 `to()` 将张量移动到 GPU 上,从而在 GPU 上更快地运行代码。
下面是优化后的代码:
```
import torch.nn as nn
class MyLinear(nn.Module):
def __init__(self, in_features, out_features):
super(MyLinear, self).__init__()
self.linears = nn.ModuleList([nn.Linear(in_features, out_features).to('cuda') for i in range(5)])
def forward(self, x):
chunks = torch.chunk(x, 5, dim=1)
chunks = torch.stack(chunks, dim=0).to('cuda')
weights = torch.stack([linear.weight.to('cuda') for linear in self.linears], dim=0)
outputs = torch.matmul(chunks, weights.transpose(1, 2)).reshape(x.shape[0], -1)
return outputs
```
此代码在创建每个线性层时使用 `.to('cuda')` 将其权重张量移动到 GPU 上。在 `forward()` 方法中,使用 `.to('cuda')` 将 `chunks` 张量和 `weights` 张量移动到 GPU 上,然后使用 `torch.matmul()` 进行矩阵乘法运算,并使用 `.reshape()` 将输出张量重新变形为二维张量,最后将其返回。
优化这段代码来提高运行速度:import torch.nn as nn class MyLinear(nn.Module): def __init__(self, in_features, out_features): super(MyLinear, self).__init__() self.linears = nn.ModuleList([nn.Linear(in_features, out_features) for i in range(5)]) def forward(self, x): chunks = torch.chunk(x, 5, dim=1) outputs = [linear(chunk) for linear, chunk in zip(self.linears, chunks)] return torch.cat(outputs, dim=1)
可以使用 PyTorch 内置的函数 `torch.matmul()` 和 `torch.transpose()` 来优化代码,从而提高运行速度。
下面是优化后的代码:
```
import torch.nn as nn
class MyLinear(nn.Module):
def __init__(self, in_features, out_features):
super(MyLinear, self).__init__()
self.linears = nn.ModuleList([nn.Linear(in_features, out_features) for i in range(5)])
def forward(self, x):
chunks = torch.chunk(x, 5, dim=1)
chunks = torch.stack(chunks, dim=0)
weights = torch.stack([linear.weight for linear in self.linears], dim=0)
outputs = torch.matmul(chunks, weights.transpose(1, 2)).reshape(x.shape[0], -1)
return outputs
```
此代码使用 `torch.chunk()` 将输入张量 `x` 沿着维度 `1` 分成 $5$ 个张量,然后使用 `torch.stack()` 将它们沿着新的维度 `0` 拼接在一起。接着使用 `torch.stack()` 将每个线性层的权重张量沿着新的维度 `0` 拼接在一起,然后使用 `torch.matmul()` 进行矩阵乘法运算,将 `chunks` 张量与 `weights` 张量的转置张量相乘,并将结果沿着维度 `1` 和 `2` 进行转置。最后使用 `torch.reshape()` 将输出张量重新变形为二维张量,得到最终的输出张量。
相关推荐
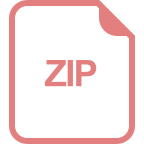
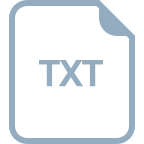










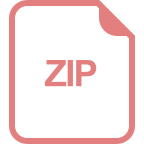
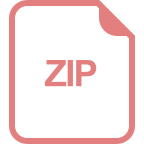
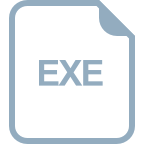