用java来实现以下内容:通讯录管理系统 1.通过java模拟链表来实现 2.实现来简单的增删改查 3.实现了通过文件来保存通讯录的信息 4.实现自定义异常来提示错误(就是想用以下自定义异常) 5.可以通过姓名和电话号码的字串来查找 6.保证了电话号码的唯一性
时间: 2024-03-17 22:45:30 浏览: 15
好的,我可以帮您解答这个问题。通讯录管理系统可以用Java语言通过链表来实现,以下是代码示例:
首先,定义一个联系人类 ContactPerson,包含姓名和电话号码两个属性:
```java
public class ContactPerson {
private String name;
private String phoneNumber;
public ContactPerson(String name, String phoneNumber) {
this.name = name;
this.phoneNumber = phoneNumber;
}
// getter and setter methods
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPhoneNumber() {
return phoneNumber;
}
public void setPhoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
}
}
```
接下来,定义一个链表节点类 ListNode,包含一个 ContactPerson 对象和下一个节点的引用:
```java
public class ListNode {
private ContactPerson contactPerson;
private ListNode next;
public ListNode(ContactPerson contactPerson) {
this.contactPerson = contactPerson;
}
// getter and setter methods
public ContactPerson getContactPerson() {
return contactPerson;
}
public void setContactPerson(ContactPerson contactPerson) {
this.contactPerson = contactPerson;
}
public ListNode getNext() {
return next;
}
public void setNext(ListNode next) {
this.next = next;
}
}
```
然后,定义一个链表类 LinkedList,实现增删改查的方法:
```java
public class LinkedList {
private ListNode head;
public void addContactPerson(ContactPerson contactPerson) {
if (head == null) {
head = new ListNode(contactPerson);
} else {
ListNode current = head;
while (current.getNext() != null) {
current = current.getNext();
}
current.setNext(new ListNode(contactPerson));
}
}
public void removeContactPerson(String name) throws ContactPersonNotFoundException {
if (head == null) {
throw new ContactPersonNotFoundException("通讯录为空,无法删除联系人!");
}
if (head.getContactPerson().getName().equals(name)) {
head = head.getNext();
} else {
ListNode current = head;
while (current.getNext() != null && !current.getNext().getContactPerson().getName().equals(name)) {
current = current.getNext();
}
if (current.getNext() == null) {
throw new ContactPersonNotFoundException("联系人不存在,无法删除!");
}
current.setNext(current.getNext().getNext());
}
}
public void updateContactPerson(ContactPerson contactPerson) throws ContactPersonNotFoundException {
if (head == null) {
throw new ContactPersonNotFoundException("通讯录为空,无法更新联系人!");
}
ListNode current = head;
while (current != null) {
if (current.getContactPerson().getName().equals(contactPerson.getName())) {
current.setContactPerson(contactPerson);
return;
}
current = current.getNext();
}
throw new ContactPersonNotFoundException("联系人不存在,无法更新!");
}
public ContactPerson findContactPersonByName(String name) throws ContactPersonNotFoundException {
if (head == null) {
throw new ContactPersonNotFoundException("通讯录为空,无法查找联系人!");
}
ListNode current = head;
while (current != null) {
if (current.getContactPerson().getName().contains(name)) {
return current.getContactPerson();
}
current = current.getNext();
}
throw new ContactPersonNotFoundException("联系人不存在,无法查找!");
}
public ContactPerson findContactPersonByPhoneNumber(String phoneNumber) throws ContactPersonNotFoundException {
if (head == null) {
throw new ContactPersonNotFoundException("通讯录为空,无法查找联系人!");
}
ListNode current = head;
while (current != null) {
if (current.getContactPerson().getPhoneNumber().equals(phoneNumber)) {
return current.getContactPerson();
}
current = current.getNext();
}
throw new ContactPersonNotFoundException("联系人不存在,无法查找!");
}
}
```
其中,ContactPersonNotFoundException 是自定义的异常类,用于提示错误信息:
```java
public class ContactPersonNotFoundException extends Exception {
public ContactPersonNotFoundException(String message) {
super(message);
}
}
```
最后,我们可以通过文件来保存通讯录的信息。例如,将通讯录保存到 contacts.txt 文件中:
```java
public void saveToFile(String fileName) throws IOException {
File file = new File(fileName);
if (!file.exists()) {
file.createNewFile();
}
BufferedWriter out = new BufferedWriter(new FileWriter(file));
ListNode current = head;
while (current != null) {
out.write(current.getContactPerson().getName() + "," + current.getContactPerson().getPhoneNumber());
out.newLine();
current = current.getNext();
}
out.flush();
out.close();
}
```
以上就是用Java模拟链表来实现通讯录管理系统的示例代码。
相关推荐
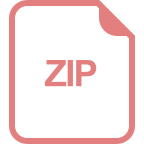














