C++ 字典存储集合
时间: 2023-09-02 20:14:54 浏览: 49
C++ 中可以使用标准库中的 `std::map` 或 `std::unordered_map` 实现字典存储集合,其中 `std::map` 使用红黑树实现,保证元素有序,而 `std::unordered_map` 使用哈希表实现,元素无序但查找速度更快。
以下是一个使用 `std::unordered_map` 存储字符串集合的示例代码:
```c++
#include <iostream>
#include <unordered_map>
#include <string>
int main() {
std::unordered_map<std::string, bool> dict;
dict["apple"] = true;
dict["banana"] = true;
dict["orange"] = true;
// 判断是否包含某个元素
if (dict.count("apple")) {
std::cout << "包含 apple" << std::endl;
}
// 遍历所有元素
for (const auto& pair : dict) {
std::cout << pair.first << std::endl;
}
return 0;
}
```
相关问题
c++ set
C++ 中的 set 是一个标准库容器,用于存储元素集合并自动按照一定的排序规则进行排序。set 中每个元素只能出现一次。
set 的实现是基于红黑树(Red-Black tree),因此插入、查找和删除元素的时间复杂度都是 O(log n)。
下面是一个简单的示例,演示了如何使用 set 存储字符串,并按字典序进行排序:
```c++
#include <set>
#include <string>
#include <iostream>
int main()
{
std::set<std::string> words;
words.insert("hello");
words.insert("world");
words.insert("set");
words.insert("container");
for (const auto& word : words)
{
std::cout << word << std::endl;
}
return 0;
}
```
在上面的代码中,我们首先创建了一个 set 对象,然后使用 insert 函数将一些字符串插入到 set 中。由于 set 自动按字典序进行排序,因此输出时会按照字典序输出元素。请注意,我们使用 const auto& 来遍历 set 中的元素,这样可以避免不必要的拷贝操作。
set 还提供了许多其他的函数,例如 find、erase 等,可以帮助我们更方便地操作集合中的元素。
c++ map和数组
C++中的map和数组是两种不同的数据结构,用于存储和操作数据。
1. 数组(Array)是一种线性数据结构,它由相同类型的元素组成,并按照一定的顺序排列。数组的特点是元素在内存中是连续存储的,可以通过索引访问元素。数组的大小在创建时就确定,并且不能动态改变大小。
2. map是C++标准库中的关联容器,也被称为字典或键值对。它是一种键值对的集合,每个键唯一对应一个值。map中的元素按照键的顺序进行排序,并且可以通过键快速查找对应的值。map内部使用红黑树实现,因此插入、删除和查找操作的时间复杂度都是O(logN)。
相关推荐
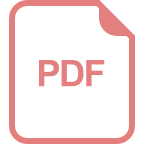
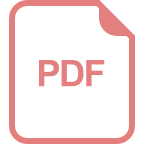
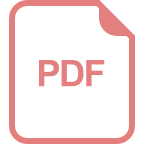
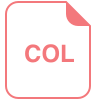











