用C语言实现字典树
时间: 2023-07-24 08:14:18 浏览: 112
好的,以下是用C语言实现字典树的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_CHILDREN 26
struct TrieNode {
int count; // 记录单词出现次数
struct TrieNode* children[MAX_CHILDREN];
};
struct Trie {
struct TrieNode* root;
};
struct TrieNode* create_node() {
struct TrieNode* node = (struct TrieNode*)malloc(sizeof(struct TrieNode));
node->count = 0;
for (int i = 0; i < MAX_CHILDREN; i++) {
node->children[i] = NULL;
}
return node;
}
void insert(struct Trie* trie, char* word) {
struct TrieNode* p = trie->root;
for (int i = 0; i < strlen(word); i++) {
int index = word[i] - 'a';
if (p->children[index] == NULL) {
p->children[index] = create_node();
}
p = p->children[index];
}
p->count++;
}
int search(struct Trie* trie, char* word) {
struct TrieNode* p = trie->root;
for (int i = 0; i < strlen(word); i++) {
int index = word[i] - 'a';
if (p->children[index] == NULL) {
return 0;
}
p = p->children[index];
}
return p->count;
}
int main() {
struct Trie trie;
trie.root = create_node();
insert(&trie, "apple");
insert(&trie, "app");
insert(&trie, "application");
int count = search(&trie, "app");
printf("单词 app 出现的次数为:%d\n", count);
return 0;
}
```
以上代码中,`create_node` 函数用于创建字典树节点,`insert` 函数用于向字典树中插入单词,`search` 函数用于在字典树中查找单词出现的次数。`main` 函数则测试了该字典树在插入单词 `"apple"`、`"app"`、`"application"` 后查找单词 `"app"` 出现的次数的结果。
阅读全文
相关推荐
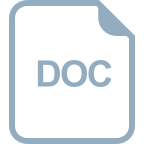
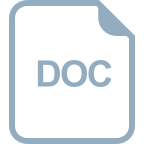


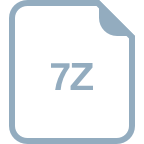
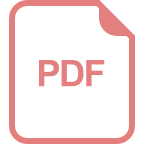
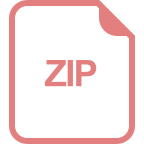
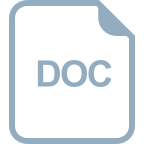
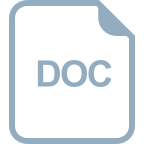



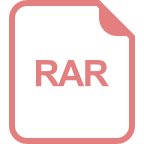
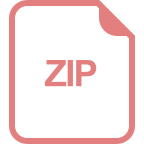
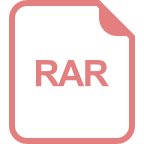
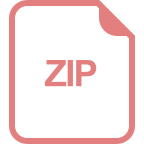