C语言字典树词频统计
时间: 2023-07-01 22:17:08 浏览: 66
好的,下面是C语言实现的字典树词频统计代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAX_WORD_LEN 100 // 单词最大长度
// 字典树节点结构体
typedef struct TrieNode {
int count; // 记录单词出现次数
struct TrieNode* next[26]; // 指向下一个字符的指针数组
} TrieNode;
// 创建新的字典树节点
TrieNode* newTrieNode() {
TrieNode* node = (TrieNode*)malloc(sizeof(TrieNode));
node->count = 0;
memset(node->next, 0, sizeof(node->next));
return node;
}
// 将单词插入字典树
void insertWord(TrieNode* root, char* word) {
TrieNode* node = root;
int len = strlen(word);
for (int i = 0; i < len; i++) {
int index = tolower(word[i]) - 'a'; // 转换为小写字母后计算下标
if (node->next[index] == NULL) {
node->next[index] = newTrieNode();
}
node = node->next[index];
}
node->count++;
}
// 统计单词出现次数
int countWords(TrieNode* root, char* word) {
TrieNode* node = root;
int len = strlen(word);
for (int i = 0; i < len; i++) {
int index = tolower(word[i]) - 'a'; // 转换为小写字母后计算下标
if (node->next[index] == NULL) {
return 0;
}
node = node->next[index];
}
return node->count;
}
int main() {
TrieNode* root = newTrieNode();
char filename[100];
printf("请输入要统计的文本文件名:");
scanf("%s", filename);
FILE* fp = fopen(filename, "r");
if (fp == NULL) {
printf("文件打开失败!\n");
return 0;
}
char word[MAX_WORD_LEN];
while (fscanf(fp, "%s", word) != EOF) {
insertWord(root, word);
}
fclose(fp);
printf("请输入要查询的单词(输入quit结束查询):\n");
while (1) {
scanf("%s", word);
if (strcmp(word, "quit") == 0) {
break;
}
printf("%s: %d\n", word, countWords(root, word));
}
return 0;
}
```
这份代码可以读取一个文本文件,将其中的单词插入字典树中,并且可以查询某个单词在文本中出现的次数。在插入单词和查询单词时,会将单词转换为小写字母后再进行操作,这样可以避免大小写不同的单词被算作不同的单词。
相关推荐
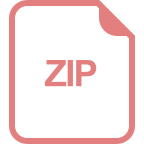














