c语言字典trie树代码实现
时间: 2023-08-24 19:18:29 浏览: 78
以下是一个简单的 C 语言字典 Trie 树的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WORD_LEN 100
typedef struct TrieNode {
char value;
struct TrieNode *children[26];
int is_end;
} TrieNode;
TrieNode *createNode(char value) {
TrieNode *node = (TrieNode *) malloc(sizeof(TrieNode));
node->value = value;
for (int i = 0; i < 26; i++) {
node->children[i] = NULL;
}
node->is_end = 0;
return node;
}
void insert(TrieNode *root, char *word) {
TrieNode *curr = root;
int len = strlen(word);
for (int i = 0; i < len; i++) {
int index = word[i] - 'a';
if (curr->children[index] == NULL) {
curr->children[index] = createNode(word[i]);
}
curr = curr->children[index];
}
curr->is_end = 1;
}
int search(TrieNode *root, char *word) {
TrieNode *curr = root;
int len = strlen(word);
for (int i = 0; i < len; i++) {
int index = word[i] - 'a';
if (curr->children[index] == NULL) {
return 0;
}
curr = curr->children[index];
}
return curr->is_end;
}
int main() {
TrieNode *root = createNode('\0');
char word[MAX_WORD_LEN];
int choice = 0;
do {
printf("1. Insert Word\n");
printf("2. Search Word\n");
printf("3. Exit\n");
printf("Enter Choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Enter Word to Insert: ");
scanf("%s", word);
insert(root, word);
break;
case 2:
printf("Enter Word to Search: ");
scanf("%s", word);
if (search(root, word)) {
printf("%s is present in the dictionary.\n", word);
} else {
printf("%s is not present in the dictionary.\n", word);
}
break;
case 3:
printf("Exiting...\n");
break;
default:
printf("Invalid Choice!\n");
break;
}
} while (choice != 3);
return 0;
}
```
该实现中使用了一个 TrieNode 结构体来表示 Trie 树中的每个节点,其中包含了节点的值,子节点指针数组和一个标志位,用于指示该节点是否为单词的结尾。
在插入单词时,从根节点开始遍历 Trie 树,如果当前节点的相应子节点为空,则新建一个节点并将其作为当前节点的相应子节点。最后将单词的结尾节点的标志位设置为 1。
在查找单词时,同样从根节点开始遍历 Trie 树,如果当前节点的相应子节点为空,则说明该单词不存在于 Trie 树中。如果单词的最后一个字符所在的节点的标志位为 1,则说明该单词存在于 Trie 树中。
该实现中还包含了一个简单的命令行界面,用于接收用户的输入并执行相应的操作。
相关推荐
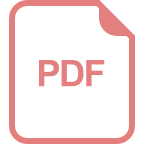












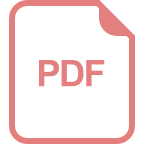