c语言构造trie
时间: 2023-06-21 14:20:00 浏览: 65
Trie,也叫字典树或前缀树,是一种树形数据结构,用于高效地存储和检索字符串数据集。下面是一个简单的 C 语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define ALPHABET_SIZE 26
// Trie 节点结构体
struct TrieNode {
struct TrieNode *children[ALPHABET_SIZE];
int is_end_of_word;
};
// 创建一个新的 Trie 节点
struct TrieNode *create_node() {
struct TrieNode *node = (struct TrieNode *)malloc(sizeof(struct TrieNode));
node->is_end_of_word = 0;
for (int i = 0; i < ALPHABET_SIZE; i++) {
node->children[i] = NULL;
}
return node;
}
// 将字符串插入 Trie 树中
void insert(struct TrieNode *root, char *key) {
struct TrieNode *node = root;
int len = strlen(key);
for (int i = 0; i < len; i++) {
int index = key[i] - 'a';
if (!node->children[index]) {
node->children[index] = create_node();
}
node = node->children[index];
}
node->is_end_of_word = 1;
}
// 在 Trie 树中查找字符串
int search(struct TrieNode *root, char *key) {
struct TrieNode *node = root;
int len = strlen(key);
for (int i = 0; i < len; i++) {
int index = key[i] - 'a';
if (!node->children[index]) {
return 0;
}
node = node->children[index];
}
return (node && node->is_end_of_word);
}
int main() {
struct TrieNode *root = create_node();
insert(root, "hello");
insert(root, "world");
insert(root, "trie");
printf("%d\n", search(root, "hello"));
printf("%d\n", search(root, "world"));
printf("%d\n", search(root, "trie"));
printf("%d\n", search(root, "hi"));
return 0;
}
```
这个实现只考虑了小写字母,如果需要支持更多字符,需要相应地修改 `ALPHABET_SIZE` 和字符转换的方式。
相关推荐
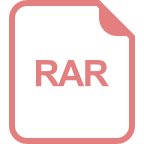
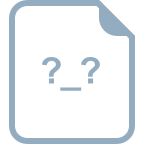














