C++鼠标连点器代码通过识别图片实现在其他应用连点
时间: 2024-03-17 08:41:19 浏览: 115
要实现通过识别图片来进行鼠标连点,需要借助第三方库(如OpenCV),并使用模板匹配来识别图片。以下是一个示例代码,可以在指定的窗口中通过识别图片来进行鼠标连点:
```c++
#include <Windows.h>
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
// 获取窗口句柄
HWND hwnd = FindWindow(NULL, "窗口标题");
// 加载模板图片
Mat imgTemplate = imread("模板图片路径");
// 设置阈值
double thresholdValue = 0.8;
// 设置点击次数
int clickCount = 10;
// 获取窗口大小
RECT rect;
GetWindowRect(hwnd, &rect);
int width = rect.right - rect.left;
int height = rect.bottom - rect.top;
// 创建屏幕截图
HDC hdc = GetDC(NULL);
HDC hdcMem = CreateCompatibleDC(hdc);
HBITMAP hBitmap = CreateCompatibleBitmap(hdc, width, height);
SelectObject(hdcMem, hBitmap);
BitBlt(hdcMem, 0, 0, width, height, hdc, rect.left, rect.top, SRCCOPY);
ReleaseDC(NULL, hdc);
// 循环点击
for (int i = 0; i < clickCount; i++)
{
// 在屏幕截图中查找模板图片
Mat imgSrc(height, width, CV_8UC4);
GetBitmapBits(hBitmap, width * height * 4, imgSrc.data);
Mat imgResult;
matchTemplate(imgSrc, imgTemplate, imgResult, TM_CCOEFF_NORMED);
Point maxLoc;
minMaxLoc(imgResult, NULL, NULL, NULL, &maxLoc);
if (imgResult.at<float>(maxLoc.y, maxLoc.x) >= thresholdValue)
{
// 计算点击位置
int x = maxLoc.x + imgTemplate.cols / 2 + rect.left;
int y = maxLoc.y + imgTemplate.rows / 2 + rect.top;
// 移动鼠标
SetCursorPos(x, y);
// 模拟左键点击
mouse_event(MOUSEEVENTF_LEFTDOWN, x, y, 0, 0);
mouse_event(MOUSEEVENTF_LEFTUP, x, y, 0, 0);
// 等待一段时间
Sleep(100);
}
}
// 释放资源
DeleteObject(hBitmap);
DeleteDC(hdcMem);
return 0;
}
```
在代码中,需要将“窗口标题”替换为要进行连点的窗口的标题,将“模板图片路径”替换为要识别的模板图片的路径。同时,需要使用OpenCV库中的函数来进行模板匹配,使用Windows API中的函数来进行屏幕截图和鼠标操作。其余代码与前面的示例类似。
需要注意的是,通过识别图片进行鼠标连点可能会受到一些干扰,如窗口大小、分辨率、图片相似度等因素。同时,使用自动化程序进行连点可能会违反某些应用程序的使用协议,应谨慎使用。
阅读全文
相关推荐



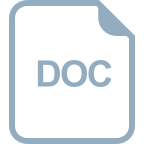
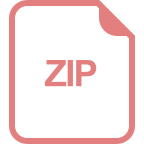
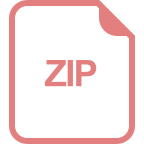
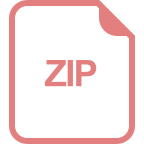
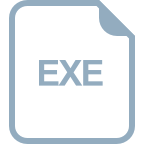
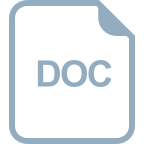