java根据字段拆分list
时间: 2023-09-01 16:11:41 浏览: 49
可以使用Java 8中的stream和map方法来实现。具体的实现代码如下:
```
public static List<String> splitListByField(List<?> list, String fieldName) {
return list.stream()
.map(obj -> {
try {
Field field = obj.getClass().getDeclaredField(fieldName);
field.setAccessible(true);
return field.get(obj).toString();
} catch (NoSuchFieldException | IllegalAccessException e) {
e.printStackTrace();
}
return null;
})
.filter(Objects::nonNull)
.collect(Collectors.toList());
}
```
示例用法:
```
class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
List<Person> personList = Arrays.asList(
new Person("张三", 20),
new Person("李四", 22),
new Person("王五", 25)
);
List<String> nameList = splitListByField(personList, "name");
List<String> ageList = splitListByField(personList, "age");
```
调用splitListByField方法,并传入需要拆分的list和字段名称即可得到拆分后的结果,在本例中,得到的nameList和ageList分别为:
```
["张三", "李四", "王五"]
[20, 22, 25]
```
相关推荐
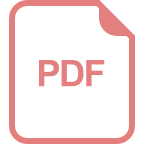
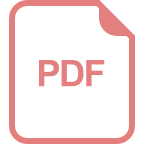















