页面置换算法LRU算法c代码
时间: 2023-04-07 19:00:48 浏览: 126
以下是LRU算法的C代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define CACHE_SIZE 4
typedef struct node {
int data;
struct node* next;
} Node;
Node* head = NULL;
int cache[CACHE_SIZE] = {0};
int cache_count = 0;
void print_cache() {
printf("Cache: ");
for (int i = 0; i < CACHE_SIZE; i++) {
printf("%d ", cache[i]);
}
printf("\n");
}
void add_to_cache(int page) {
if (cache_count < CACHE_SIZE) {
cache[cache_count++] = page;
} else {
Node* current = head;
while (current->next != NULL) {
current = current->next;
}
int oldest_page = current->data;
current->data = page;
for (int i = 0; i < CACHE_SIZE; i++) {
if (cache[i] == oldest_page) {
cache[i] = page;
break;
}
}
}
}
void access_page(int page) {
for (int i = 0; i < CACHE_SIZE; i++) {
if (cache[i] == page) {
printf("Page %d is already in cache.\n", page);
return;
}
}
printf("Page %d is not in cache.\n", page);
add_to_cache(page);
print_cache();
}
int main() {
head = (Node*)malloc(sizeof(Node));
head->data = -1;
head->next = NULL;
access_page(1);
access_page(2);
access_page(3);
access_page(4);
access_page(1);
access_page(2);
access_page(5);
access_page(1);
access_page(2);
access_page(3);
access_page(4);
access_page(5);
return 0;
}
```
这段代码实现了LRU算法的缓存置换策略。当访问一个页面时,如果该页面已经在缓存中,则直接返回;否则,将该页面加入缓存中,并将最久未被访问的页面替换掉。
相关推荐
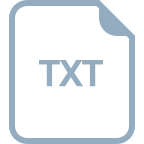














