fastapi 中 使用 queue
时间: 2023-09-13 20:05:56 浏览: 372
在 FastAPI 中使用队列可以通过 Python 内置的 `queue` 模块实现。具体步骤如下:
1. 导入 `queue` 模块:
```python
import queue
```
2. 创建一个队列对象:
```python
my_queue = queue.Queue()
```
3. 向队列中添加元素:
```python
my_queue.put('item')
```
4. 从队列中获取元素:
```python
item = my_queue.get()
```
如果队列为空,`get()` 方法会一直阻塞直到队列中有元素。
5. 可以通过 `task` 和 `asyncio` 模块实现异步处理队列:
```python
import asyncio
async def consume_queue():
while True:
item = await asyncio.to_thread(my_queue.get)
# do something with item
async def produce_queue():
while True:
item = await produce_item()
await asyncio.to_thread(my_queue.put, item)
```
在上面的例子中,`consume_queue()` 函数将在事件循环中等待并消费队列中的元素,而 `produce_queue()` 函数则会生产元素并将它们放入队列中。
当你使用 FastAPI 时,你可以将上述代码嵌入到你的视图函数中,用于异步处理请求。
阅读全文
相关推荐
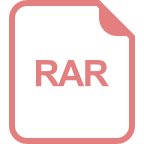
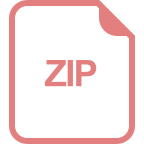
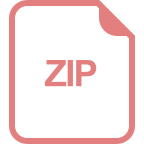








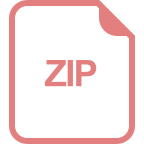
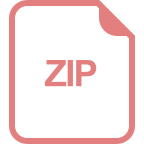
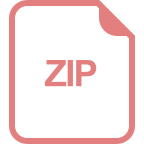
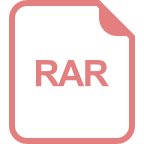
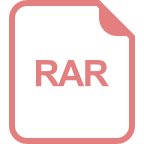
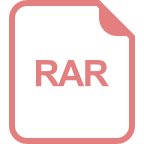
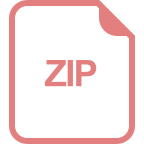
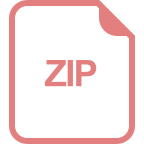