java.util.Date 通过string "10" 加10分钟
时间: 2024-02-22 20:56:15 浏览: 62
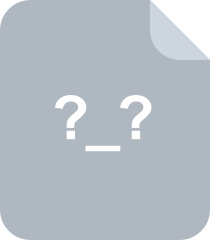
按每10分钟计算数据的次数
可以使用 `java.text.SimpleDateFormat` 类来将字符串类型的时间转换成 `java.util.Date` 类型,并使用 `java.util.Calendar` 类来实现时间的加减操作,以下是将字符串 "10" 加上 10 分钟的示例代码:
```java
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
public class DateExample {
public static void main(String[] args) throws ParseException {
// 定义时间格式
SimpleDateFormat sdf = new SimpleDateFormat("HH:mm:ss");
// 将字符串转换成 Date 类型
Date date = sdf.parse("10:00:00");
// 使用 Calendar 类来实现时间的加减操作
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
calendar.add(Calendar.MINUTE, 10);
Date newDate = calendar.getTime();
// 将新的日期转换成字符串
String newDateString = sdf.format(newDate);
// 输出结果
System.out.println("原时间:" + sdf.format(date));
System.out.println("加上10分钟后的时间:" + newDateString);
}
}
```
在这个示例代码中,我们首先定义了时间格式为 "HH:mm:ss" 的 `SimpleDateFormat` 对象 `sdf`,然后使用 `sdf.parse()` 方法将字符串 "10:00:00" 转换成 `java.util.Date` 类型的时间 `date`。接着,我们使用 `Calendar.getInstance()` 方法创建一个 `Calendar` 对象 `calendar`,并将 `date` 设置为 `calendar` 的时间。然后,我们使用 `calendar.add()` 方法将时间加上 10 分钟,并使用 `calendar.getTime()` 方法将 `calendar` 转换成 `java.util.Date` 类型的时间 `newDate`。最后,我们使用 `sdf.format()` 方法将 `newDate` 转换成字符串类型的时间 `newDateString`,并输出结果。
需要注意的是,这个示例代码中使用的时间格式为 "HH:mm:ss",如果需要使用其他的时间格式,需要相应地修改 `SimpleDateFormat` 对象的格式。另外,这里的时间加减操作是以分钟为单位,如果需要以其他的时间单位进行加减操作,需要调用相应的 `Calendar` 方法。
阅读全文
相关推荐
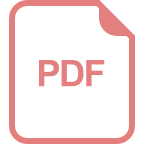

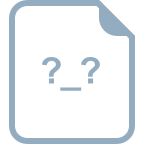













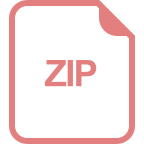
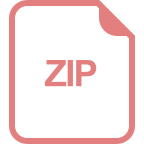