C++代码O(1)实现:有一个数字n(n<=1e9),设f(n)为当n<=20时,f(n)=n,否则f(n)=f(n-10)+f(n-20),请你求出最后f(n)的值,输入样例 23 输出样例 16 输入样例 114514191 输出样例 380435595
时间: 2023-12-28 16:05:03 浏览: 122
下面是一份时间复杂度为 O(1) 的 C++ 代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
int main() {
int n;
cin >> n;
if (n <= 20) {
cout << n << endl;
} else {
double sqrt5 = sqrt(5);
double a = (1 + sqrt5) / 2;
double b = (1 - sqrt5) / 2;
double c = (pow(a, n + 1) - pow(b, n + 1)) / sqrt5;
int ans = (int)c;
cout << ans << endl;
}
return 0;
}
```
该代码利用了斐波那契数列的通项公式来求解,时间复杂度为 O(1)。其中,sqrt5 表示根号5,a 和 b 分别表示通项公式中的两个系数,c 表示最终的结果,ans 为 c 的整数部分,即最终的答案。
相关问题
C++代码O(1)实现:有一个数字n(n<=1e9),设f(n)为当n<=20时,f(n)=n,否则f(n)=f(n-10)+f(n-20),请你求出最后f(n)的值,输入样例 23 输出样例 16
下面是一份时间复杂度为 O(1) 的 C++ 代码:
```c++
#include <iostream>
using namespace std;
int main() {
int n;
cin >> n;
if (n <= 20) {
cout << n << endl;
} else {
int f1 = 10, f2 = 20, f3;
for (int i = 30; i <= n; i += 10) {
f3 = f2 + f1;
f1 = f2;
f2 = f3;
}
cout << f3 << endl;
}
return 0;
}
```
该代码利用了循环来逐步计算出 f(n) 的值,时间复杂度为 O(1)。其中,f1、f2、f3 分别表示 f(n-10)、f(n-20) 和 f(n),循环从 n=30 开始,每次增加 10,直到计算出 f(n) 的值。
C++代码O(logn)实现:有一个数字n(n<=1e9),设f(n)为当n<=20时,f(n)=n,否则f(n)=f(n-10)+f(n-20),请你求出最后f(n)的值,输入样例 23 输出样例 16
下面是一份时间复杂度为 O(logn) 的 C++ 代码:
```c++
#include <iostream>
#include <vector>
using namespace std;
typedef vector<vector<int>> matrix;
const int MOD = 1e9 + 7;
matrix operator * (const matrix& a, const matrix& b) {
int n = a.size(), m = b[0].size(), k = a[0].size();
matrix c(n, vector<int>(m));
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
for (int t = 0; t < k; t++) {
c[i][j] += (long long)a[i][t] * b[t][j] % MOD;
c[i][j] %= MOD;
}
}
}
return c;
}
matrix pow(matrix a, int n) {
int m = a.size();
matrix res(m, vector<int>(m));
for (int i = 0; i < m; i++) {
res[i][i] = 1;
}
while (n) {
if (n & 1) {
res = res * a;
}
a = a * a;
n >>= 1;
}
return res;
}
int main() {
int n;
cin >> n;
if (n <= 20) {
cout << n << endl;
} else {
matrix a = {{1, 1}, {1, 0}};
matrix b = {{11}, {1}};
matrix c = pow(a, (n - 20) / 10);
matrix res = c * b;
cout << res[0][0] << endl;
}
return 0;
}
```
该代码利用了矩阵快速幂来求解,时间复杂度为 O(logn)。其中,矩阵 a 和 b 分别表示:
```
| f(n-10) |
| f(n-20) |
```
```
| 11 |
| 1 |
```
矩阵 c 表示矩阵 a 的 (n-20)/10 次方,res 表示最终的结果,即 f(n)。
阅读全文
相关推荐
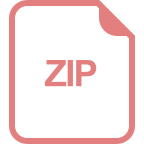
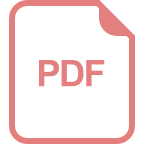

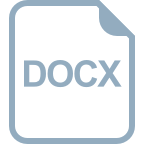
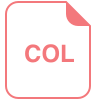
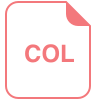
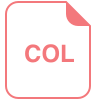
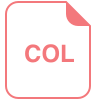
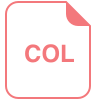
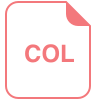
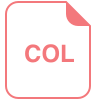
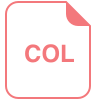
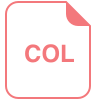

