python 数组位操作
时间: 2023-09-10 15:08:17 浏览: 47
Python中的数组可以使用numpy库中的ndarray来表示,同时,numpy库中也提供了一些位操作的函数。以下是一些常用的位操作函数:
1. bitwise_and(arr1, arr2):对两个数组进行按位与操作。
2. bitwise_or(arr1, arr2):对两个数组进行按位或操作。
3. bitwise_xor(arr1, arr2):对两个数组进行按位异或操作。
4. bitwise_not(arr):对数组进行按位取反操作。
5. left_shift(arr, shift):对数组进行左移操作。
6. right_shift(arr, shift):对数组进行右移操作。
以下是一些位操作的示例代码:
```python
import numpy as np
# 按位与
a = np.array([0b1010, 0b1100], dtype=np.uint8)
b = np.array([0b1111, 0b0011], dtype=np.uint8)
c = np.bitwise_and(a, b)
print(c) # 输出 [10 0]
# 按位或
a = np.array([0b1010, 0b1100], dtype=np.uint8)
b = np.array([0b1111, 0b0011], dtype=np.uint8)
c = np.bitwise_or(a, b)
print(c) # 输出 [15 13]
# 按位异或
a = np.array([0b1010, 0b1100], dtype=np.uint8)
b = np.array([0b1111, 0b0011], dtype=np.uint8)
c = np.bitwise_xor(a, b)
print(c) # 输出 [ 5 13]
# 按位取反
a = np.array([0b1010, 0b1100], dtype=np.uint8)
b = np.bitwise_not(a)
print(b) # 输出 [245 203]
# 左移
a = np.array([0b1010, 0b1100], dtype=np.uint8)
b = np.left_shift(a, 2)
print(b) # 输出 [40 48]
# 右移
a = np.array([0b1010, 0b1100], dtype=np.uint8)
b = np.right_shift(a, 2)
print(b) # 输出 [ 2 3]
```
相关推荐
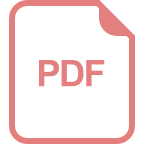
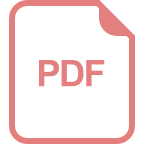
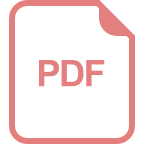
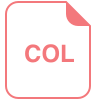
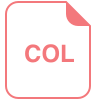
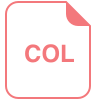
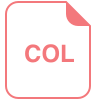
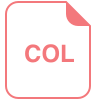









