python去除小数点后的无效0
时间: 2024-05-01 07:19:38 浏览: 58
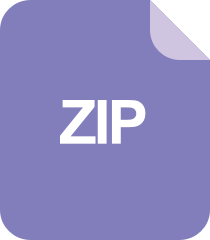
python 手写数字识别(包含小数点).zip
您可以使用Python内置函数`float()`和`rstrip()`来去除小数点后的无效0。具体操作如下:
```python
num = 3.140000
num_str = str(num) # 先将数字转化为字符串
num_without_zeros = float(num_str.rstrip('0').rstrip('.'))
print(num_without_zeros) # 输出结果为3.14
```
这个方法中,首先我们将数字转换为字符串,然后使用`rstrip()`函数去除右侧的'0'和小数点'.',最后再将数字用`float()`函数转换回来。这样就可以去掉小数点后的无效0了。
阅读全文
相关推荐
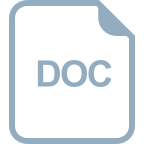
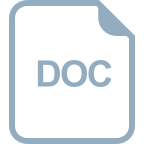
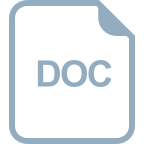
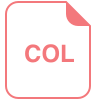
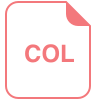
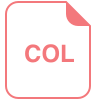
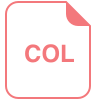
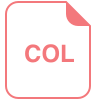
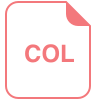



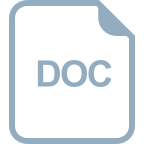
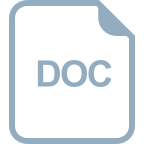
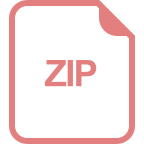
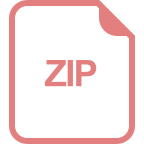