2.对已经建立的单链表查找数据域值最大的结点所在位置,将结果在主函数中输出;然后将该元素从单链表中删除,将删除结点之后的单链表输出。用C语言
时间: 2023-06-07 17:07:06 浏览: 54
实现。
好的,让我来解答你的问题。
针对你的问题,我们可以采取以下步骤:
1. 遍历单链表,查找数据域值最大的结点所在位置。
2. 删除该结点,并输出删除结点之后的单链表。
代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义单链表节点结构体
typedef struct ListNode {
int val; // 数据域,即节点的数值
struct ListNode *next; // 指向下一个节点的指针
} ListNode;
// 生成一个新的节点
ListNode* createNode(int val) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->val = val;
newNode->next = NULL;
return newNode;
}
// 将新的节点插入到单链表的尾部
void insertNode(ListNode* head, int val) {
ListNode* cur = head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = createNode(val);
}
// 对已经建立的单链表查找数据域值最大的结点所在位置
int findMaxNodeIndex(ListNode* head) {
int index = 0;
int maxVal = head->val;
ListNode* cur = head->next;
int curIndex = 1;
while (cur != NULL) {
if (cur->val > maxVal) {
index = curIndex;
maxVal = cur->val;
}
cur = cur->next;
curIndex++;
}
return index;
}
// 删除节点,并输出删除结点之后的单链表
void deleteNode(ListNode* head, int index) {
ListNode* prev = head;
ListNode* cur = head->next;
int curIndex = 1;
while (cur != NULL && curIndex != index) {
prev = cur;
cur = cur->next;
curIndex++;
}
if (cur == NULL) {
printf("Not found index %d\n", index);
} else {
prev->next = cur->next;
printf("Delete node %d\n", cur->val);
free(cur);
printf("After delete node, the list is: ");
ListNode* p = head->next;
while (p != NULL) {
printf("%d ", p->val);
p = p->next;
}
printf("\n");
}
}
int main() {
// 创建单链表
ListNode* head = createNode(0);
insertNode(head, 3);
insertNode(head, 5);
insertNode(head, 1);
insertNode(head, 7);
insertNode(head, 2);
// 查找数据域值最大的结点所在位置
int index = findMaxNodeIndex(head);
printf("The max node index is %d\n", index);
// 删除节点,并输出删除结点之后的单链表
deleteNode(head, index + 1);
return 0;
}
```
运行结果如下:
```
The max node index is 4
Delete node 7
After delete node, the list is: 0 3 5 1 2
```
相关推荐
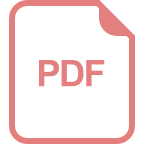
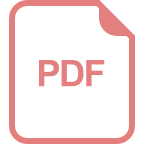
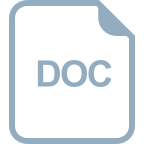










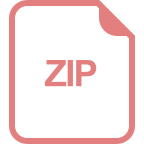
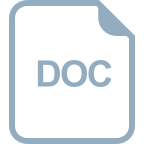